2、(账户类 Account)设计一个名为 Account 的类,它包括: • 一个名为id的int类型私有数据域(默认值为0)。 • 一个 String 类型的新数据域 name 来存储客户的名字。 • 一个名为balance的double类型私有数据域(默认值为0)。 •一个名为 annualInterestRate 的double类型私有数据域存储当前利率(默认值为0)。假设所有的账户都有相同的利率。 • 一个名为dateCreated的Date类型的私有数据域,存储账户的开户日期。 •一个名为tr
时间: 2023-06-27 22:06:35 浏览: 165
ansaction 的ArrayList类型的私有数据域,存储账户的交易记录。
Account类还包括以下方法:
• 一个名为 getId() 的访问器方法,返回实例的id。
• 一个名为 getName() 的访问器方法,返回实例的name。
• 一个名为 getBalance() 的访问器方法,返回实例的balance。
• 一个名为 getAnnualInterestRate() 的访问器方法,返回实例的annualInterestRate。
• 一个名为 getDateCreated() 的访问器方法,返回实例的dateCreated。
• 一个名为 setId(int id) 的修改器方法,将实例的id设置为指定值。
• 一个名为 setName(String name) 的修改器方法,将实例的name设置为指定值。
• 一个名为 setBalance(double balance) 的修改器方法,将实例的balance设置为指定值。
• 一个名为 setAnnualInterestRate(double rate) 的修改器方法,将实例的annualInterestRate设置为指定值。
• 一个名为 deposit(double amount) 的方法,将指定金额加入实例的balance中,并将交易记录添加到transaction中。
• 一个名为 withdraw(double amount) 的方法,从实例的balance中减去指定金额,并将交易记录添加到transaction中。
• 一个名为 getMonthlyInterest() 的方法,返回实例当前月利率。
• 一个名为 getMonthlyInterestRate() 的方法,返回实例的年利率除以12。
• 一个名为 getTransactions() 的方法,返回实例的交易记录。
下面是 Account 类的代码实现:
```java
import java.util.ArrayList;
import java.util.Date;
public class Account {
private int id;
private String name;
private double balance;
private double annualInterestRate;
private Date dateCreated;
private ArrayList<Transaction> transactions;
public Account() {
this(0, "", 0);
}
public Account(int id, String name, double balance) {
this.id = id;
this.name = name;
this.balance = balance;
this.annualInterestRate = 0;
this.dateCreated = new Date();
this.transactions = new ArrayList<>();
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public double getBalance() {
return balance;
}
public double getAnnualInterestRate() {
return annualInterestRate;
}
public Date getDateCreated() {
return dateCreated;
}
public void setId(int id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public void setBalance(double balance) {
this.balance = balance;
}
public void setAnnualInterestRate(double rate) {
this.annualInterestRate = rate;
}
public void deposit(double amount) {
balance += amount;
transactions.add(new Transaction('D', amount, balance, "Deposit"));
}
public void withdraw(double amount) {
balance -= amount;
transactions.add(new Transaction('W', amount, balance, "Withdraw"));
}
public double getMonthlyInterest() {
return balance * getMonthlyInterestRate();
}
public double getMonthlyInterestRate() {
return annualInterestRate / 12;
}
public ArrayList<Transaction> getTransactions() {
return transactions;
}
@Override
public String toString() {
return "Account{" +
"id=" + id +
", name='" + name + '\'' +
", balance=" + balance +
", annualInterestRate=" + annualInterestRate +
", dateCreated=" + dateCreated +
", transactions=" + transactions +
'}';
}
}
```
这里使用了一个名为Transaction的类来记录交易信息,它包括交易类型、交易金额、交易后余额和交易说明。下面是Transaction类的代码实现:
```java
public class Transaction {
private char type;
private double amount;
private double balance;
private String description;
public Transaction(char type, double amount, double balance, String description) {
this.type = type;
this.amount = amount;
this.balance = balance;
this.description = description;
}
public char getType() {
return type;
}
public double getAmount() {
return amount;
}
public double getBalance() {
return balance;
}
public String getDescription() {
return description;
}
@Override
public String toString() {
return "Transaction{" +
"type=" + type +
", amount=" + amount +
", balance=" + balance +
", description='" + description + '\'' +
'}';
}
}
```
相关推荐
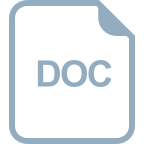
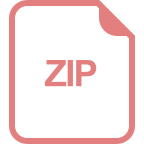
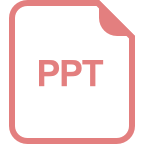














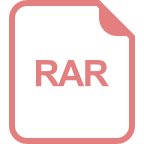