import java.util.Scanner; class TDVector { private double x; private double y; public String toString() { return "("+this.x+","+this.y+")"; } /** 你所提交的代码将被嵌在这里(替换此行) **/ } public class Main { public static void main(String[] args) { TDVector a = new TDVector(); /* 无参构造,向量的x和y默认为0 */ Scanner sc = new Scanner(System.in); double x,y,z; x = sc.nextDouble(); y = sc.nextDouble(); z = sc.nextDouble(); TDVector b = new TDVector(x, y); /* 按照参数构造向量的x和y */ TDVector c = new TDVector(b); /* 按照向量b构造向量 */ a.setY(z); System.out.println(a); System.out.println(b); System.out.println(c); c.setX(z); a = b.add(c); System.out.println(a); System.out.println("b.x="+b.getX()+" b.y="+b.getY()); sc.close(); } }
时间: 2024-01-19 07:05:04 浏览: 121
请在TDVector类中添加以下代码:
```java
public TDVector() {
this.x = 0;
this.y = 0;
}
public TDVector(double x, double y) {
this.x = x;
this.y = y;
}
public TDVector(TDVector v) {
this.x = v.x;
this.y = v.y;
}
public double getX() {
return this.x;
}
public double getY() {
return this.y;
}
public void setX(double x) {
this.x = x;
}
public void setY(double y) {
this.y = y;
}
public TDVector add(TDVector v) {
TDVector result = new TDVector();
result.x = this.x + v.x;
result.y = this.y + v.y;
return result;
}
```
主要是实现了无参构造函数、按照参数构造向量的构造函数、按照向量构造向量的构造函数、获取和设置x、y坐标的方法以及向量相加的方法。在主函数中创建了3个向量a、b、c,分别通过不同的构造函数进行初始化,然后调用相应的方法进行操作,并输出结果。
相关问题
package test; /*import java.awt.Point;*/ import java.util.Scanner; import java.util.function.DoubleToIntFunction; public class Main{ public static void main(String[] args) { Point start=new Point(); start.setX(10.0); start.setY(10.0); System.out.println("start: "+start); Point end = new Point(); // 用有参构造方法创建点(20.0, 30.0) System.out.println("end: " + end); System.out.println("Distance from start to origin: " + start.distance()); System.out.println("Distance from start to end: " + start.distance(end)); start.move(50.0, 50.0); System.out.println("start after move: " + start); end.move(start); System.out.println("end after move: " + end);} } public class Point{ private double x; private double y; public Point() { x=0.0; y=0.0; } public Point(double x,double y) { this.x=x; this.y=y; } public void setX(double x) { // 设置x坐标 this.x = x; } public void setY(double y) { // 设置y坐标 this.y = y; } public double setter() { // 获取x坐标 return x; } public double getter() { // 获取y坐标 return y; } public String toString() { return "x="+x+",y="+y; } public double distance(Point p) { double a=x-p.setter(); double b=y-p.getter(); return Math.sqrt(a*a+b*b); } public double distance() { return Math.sqrt(x*x+y*y); } public Point move(double x,double y) { this.x=x; this.y=y; return this; } public Point move(Point p) { this.x=p.setter(); this.y=p.getter(); return this; } }
这段程序是一个基于Java语言的点和向量操作的程序。它创建了一个点(Point)类,并实现了这个类的一些基本方法,例如:设置点的横纵坐标(setX、setY)、获取点的横纵坐标(getter)、求点与原点的距离(distance)、求两点间的距离(distance)、点的移动(move)等。在主函数中,程序创建了两个点对象,并调用了它们的一些方法,输出一些结果信息。在这段代码中,我们可以学习到Java语言中的对象、类、方法、参数传递等一些基本概念。
代码错误import java.util.Scanner; public class Main{ public static void main (String args[]){ Shape s=new Oval(a,b); System.out.println("The area of "+s.toString()+" is "+s.area()); System.out.println("The perimeterof "+s.toString()+" is "+s.perimeter()); }} class Shape{ abstract double area(); abstract double perimeter(); } class Oval extends Shape{ private double a; //长轴半径 private double b; //短轴半径 Oval(double a,double b){ Scanner scanner = new Scanner(System.in); this.a= scanner.nextDouble(); this.b= scanner.nextDouble(); } Oval(){ this.a=0; this.b=0; } double area(){ return Math.PI*a*b; } double perimeter(){ return 2*Math.PI*b+4*(a-b); //L=2πb+4(a-b) } public String toString() { return "Oval(a:" + this.a + ",b:" + this.b + ")"; } }
这段代码有两个问题:
1. 在创建 Oval 对象时,应该将 main 函数中的 a 和 b 作为参数传递进去,而不是从控制台读取输入。因此,Shape s=new Oval(a,b); 应该改为 Shape s=new Oval(3, 4);(3 和 4 可以替换为任意值)
2. 在 Shape 类中的 abstract 方法需要添加 abstract 关键字,因为 Shape 类是一个抽象类。
修改后的代码如下:
```java
import java.util.Scanner;
public class Main {
public static void main(String args[]) {
double a = 3; // 长轴半径
double b = 4; // 短轴半径
Shape s = new Oval(a, b);
System.out.println("The area of " + s.toString() + " is " + s.area());
System.out.println("The perimeter of " + s.toString() + " is " + s.perimeter());
}
}
abstract class Shape {
abstract double area();
abstract double perimeter();
}
class Oval extends Shape {
private double a; // 长轴半径
private double b; // 短轴半径
Oval(double a, double b) {
this.a = a;
this.b = b;
}
Oval() {
this.a = 0;
this.b = 0;
}
double area() {
return Math.PI * a * b;
}
double perimeter() {
return 2 * Math.PI * b + 4 * (a - b); // L=2πb+4(a-b)
}
public String toString() {
return "Oval(a:" + this.a + ",b:" + this.b + ")";
}
}
```
阅读全文
相关推荐
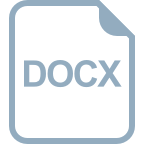
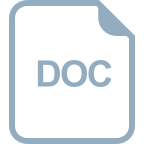














