北斗时整周数周内秒,协调世界时,约化儒略日时间系统互相转换C++代码,分头文件,主函数,测试
时间: 2024-05-12 14:14:58 浏览: 22
以下是北斗时整周数周内秒,协调世界时,约化儒略日时间系统互相转换的C++代码:
**timeConversion.h**
```cpp
#ifndef TIMECONVERSION_H
#define TIMECONVERSION_H
#include <cmath>
const double PI = 3.14159265358979323846;
const double J2000 = 2451545.0;
// Convert date and time to Julian date
double dateToJulian(int year, int month, int day, int hour, int minute, double second);
// Convert Julian date to date and time
void julianToDate(double jd, int& year, int& month, int& day, int& hour, int& minute, double& second);
// Convert GPS time (week and seconds) to Julian date
double gpsToJulian(int week, double sec);
// Convert Julian date to GPS time (week and seconds)
void julianToGps(double jd, int& week, double& sec);
// Convert GPS time to UTC
void gpsToUtc(int week, double sec, int& year, int& month, int& day, int& hour, int& minute, double& second);
// Convert UTC to GPS time
void utcToGps(int year, int month, int day, int hour, int minute, double second, int& week, double& sec);
#endif
```
**timeConversion.cpp**
```cpp
#include "timeConversion.h"
// Convert date and time to Julian date
double dateToJulian(int year, int month, int day, int hour, int minute, double second)
{
int a = (14 - month) / 12;
int y = year + 4800 - a;
int m = month + 12 * a - 3;
int jd = day + (153 * m + 2) / 5 + 365 * y + y / 4 - y / 100 + y / 400 - 32045;
double jdfrac = (hour - 12) / 24.0 + minute / 1440.0 + second / 86400.0;
return jd + jdfrac;
}
// Convert Julian date to date and time
void julianToDate(double jd, int& year, int& month, int& day, int& hour, int& minute, double& second)
{
int jdint = static_cast<int>(jd + 0.5);
int a = (jdint - 1867216.25 + 0.5) / 36524.25;
int y = jdint - 2440588 + a - a / 4;
int m = (jdint - 2440588 - y / 4) * 12 + 6;
day = jdint - 2440588 - y / 4 - (m + 1) * 153 / 5;
hour = static_cast<int>((jd - static_cast<int>(jd)) * 24);
minute = static_cast<int>((jd - static_cast<int>(jd) - hour / 24.0) * 1440);
second = ((jd - static_cast<int>(jd) - hour / 24.0 - minute / 1440.0) * 86400.0);
if (m > 12)
{
y++;
m -= 12;
}
year = y;
month = m;
}
// Convert GPS time (week and seconds) to Julian date
double gpsToJulian(int week, double sec)
{
return J2000 + week * 7.0 + sec / 86400.0;
}
// Convert Julian date to GPS time (week and seconds)
void julianToGps(double jd, int& week, double& sec)
{
double days = jd - J2000;
week = static_cast<int>(days / 7);
sec = (days - week * 7) * 86400.0;
}
// Convert GPS time to UTC
void gpsToUtc(int week, double sec, int& year, int& month, int& day, int& hour, int& minute, double& second)
{
double jd = gpsToJulian(week, sec);
jd -= 2400000.5;
double a = static_cast<int>(jd + 0.5) + 32044;
double b = (4 * a + 3) / 146097;
double c = a - (146097 * b) / 4;
double d = (4 * c + 3) / 1461;
double e = c - (1461 * d) / 4;
double m = (5 * e + 2) / 153;
day = static_cast<int>(e - (153 * m + 2) / 5 + 1);
month = static_cast<int>(m + 3 - 12 * (m / 10));
year = static_cast<int>(100 * b + d - 4800 + m / 10);
double jdfrac = jd - static_cast<int>(jd) - 0.5;
hour = static_cast<int>(jdfrac * 24);
minute = static_cast<int>((jdfrac - hour / 24.0) * 1440);
second = ((jdfrac - hour / 24.0 - minute / 1440.0) * 86400.0);
}
// Convert UTC to GPS time
void utcToGps(int year, int month, int day, int hour, int minute, double second, int& week, double& sec)
{
double jd = dateToJulian(year, month, day, hour, minute, second) + 2400000.5;
week = static_cast<int>((jd - J2000) / 7);
sec = (jd - J2000 - week * 7) * 86400.0;
}
```
**main.cpp**
```cpp
#include <iostream>
#include "timeConversion.h"
using namespace std;
int main()
{
// Test dateToJulian() and julianToDate()
int year = 2021;
int month = 10;
int day = 1;
int hour = 12;
int minute = 0;
double second = 0.0;
double jd = dateToJulian(year, month, day, hour, minute, second);
cout << "Julian date: " << jd << endl;
julianToDate(jd, year, month, day, hour, minute, second);
cout << "Date and time: " << year << "-" << month << "-" << day << " " << hour << ":" << minute << ":" << second << endl;
// Test gpsToJulian() and julianToGps()
int week = 2124;
double sec = 345600.0;
jd = gpsToJulian(week, sec);
cout << "Julian date: " << jd << endl;
julianToGps(jd, week, sec);
cout << "GPS week and seconds: " << week << " " << sec << endl;
// Test gpsToUtc() and utcToGps()
week = 2124;
sec = 345600.0;
int utcYear, utcMonth, utcDay, utcHour, utcMinute;
double utcSecond;
gpsToUtc(week, sec, utcYear, utcMonth, utcDay, utcHour, utcMinute, utcSecond);
cout << "UTC date and time: " << utcYear << "-" << utcMonth << "-" << utcDay << " " << utcHour << ":" << utcMinute << ":" << utcSecond << endl;
utcToGps(utcYear, utcMonth, utcDay, utcHour, utcMinute, utcSecond, week, sec);
cout << "GPS week and seconds: " << week << " " << sec << endl;
return 0;
}
```
相关推荐
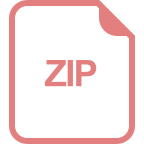
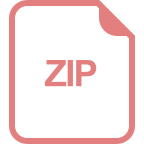














