使用微信小程序开发可视化效果 使用echarts和ec-canvas标签实现,数据的结构为 // 定义数据结构 let data = { '地区': { '广东': { '未上过学': { '合计': 100, '男': 50, '女': 50 }, '小学': { '合计': 400, '男': 200, '女': 200 }, // ...其他受教育程度 }, '广西': { '未上过学': { '合计': 50, '男': 25, '女': 25 }, '小学': { '合计': 500, '男': 250, '女': 250 }, // ... } } }; 实现一个柱状图当中的折柱混合,柱状图根据性别和地区两个指标分不同颜色,比如广东男为颜色1,广东女为颜色2,广西男为颜色3,广西女为颜色4,柱子为同一个地区(男和女)堆叠在同一个柱子,不同地区的则多一个Y轴,比如广东男和广东女在同一个柱子,折线的不同地区有多个折线用不同颜色区分,比如广西一个折线,广东一个折线,每一个点为(这个地区的这个学历)男女人数的合计数,y轴为人数,x轴为受教育程度,请写出示例代码
时间: 2024-02-05 20:04:54 浏览: 19
以下是一个使用 echarts 和 ec-canvas 标签实现的柱状图当中的折柱混合的示例代码,数据结构为题目中给出的格式:
```html
<view class="echarts">
<ec-canvas id="mychart-dom-bar" canvas-id="mychart-bar" ec="{{ ec }}"></ec-canvas>
</view>
```
```javascript
import * as echarts from '../../components/ec-canvas/echarts';
Page({
data: {
ec: {
lazyLoad: true
},
data: {
'地区': {
'广东': {
'未上过学': {
'合计': 100,
'男': 50,
'女': 50
},
'小学': {
'合计': 400,
'男': 200,
'女': 200
},
// ...其他受教育程度
},
'广西': {
'未上过学': {
'合计': 50,
'男': 25,
'女': 25
},
'小学': {
'合计': 500,
'男': 250,
'女': 250
},
// ...
}
}
},
},
onLoad: function () {
this.initChart();
},
initChart: function () {
const that = this;
const data = this.data.data;
this.selectComponent('#mychart-dom-bar').init((canvas, width, height, dpr) => {
const chart = echarts.init(canvas, null, {
width: width,
height: height,
devicePixelRatio: dpr
});
canvas.setChart(chart);
const colorList = ['#5470c6', '#91cc75', '#fac858', '#ee6666', '#73c0de', '#3ba272', '#fc8452', '#9a60b4', '#ea7ccc'];
const seriesData = [];
const yAxisData = [];
const xAxisData = [];
Object.keys(data['地区']).forEach((region, index) => {
const maleData = [];
const femaleData = [];
Object.keys(data['地区'][region]).forEach((education, idx) => {
const maleCount = data['地区'][region][education]['男'];
const femaleCount = data['地区'][region][education]['女'];
const totalCount = data['地区'][region][education]['合计'];
if (index === 0 && idx === 0) {
Object.keys(data['地区'][region][education]).forEach((key) => {
if (key !== '合计') {
xAxisData.push(key);
}
});
}
maleData.push(maleCount);
femaleData.push(femaleCount);
});
yAxisData.push(region);
seriesData.push({
name: region + '男',
type: 'bar',
stack: '总量',
data: maleData,
itemStyle: {
normal: {
color: colorList[index * 2]
}
}
});
seriesData.push({
name: region + '女',
type: 'bar',
stack: '总量',
data: femaleData,
itemStyle: {
normal: {
color: colorList[index * 2 + 1]
}
}
});
});
Object.keys(data['地区']).forEach((region, index) => {
const lineData = [];
Object.keys(data['地区'][region]).forEach((education, idx) => {
const totalCount = data['地区'][region][education]['合计'];
lineData.push(totalCount);
});
seriesData.push({
name: region,
type: 'line',
data: lineData,
yAxisIndex: index,
itemStyle: {
normal: {
color: colorList[index + 2]
}
}
});
});
chart.setOption({
tooltip: {
trigger: 'axis',
axisPointer: {
type: 'shadow'
}
},
legend: {
data: yAxisData.concat(Object.keys(data['地区'])),
textStyle: {
fontSize: 10
}
},
grid: {
left: '3%',
right: '4%',
bottom: '3%',
containLabel: true
},
xAxis: [{
type: 'category',
data: xAxisData,
axisTick: {
alignWithLabel: true
}
}],
yAxis: [{
type: 'value',
name: '人数',
position: 'left',
axisLine: {
show: false
},
axisTick: {
show: false
},
splitLine: {
show: false
}
}, {
type: 'value',
name: '人数',
position: 'right',
offset: 0,
axisLine: {
show: false
},
axisTick: {
show: false
},
splitLine: {
show: false
}
}, {
type: 'value',
name: '人数',
position: 'right',
offset: 60,
axisLine: {
show: false
},
axisTick: {
show: false
},
splitLine: {
show: false
}
}],
series: seriesData
});
return chart;
});
},
});
```
代码中的 `initChart()` 方法实现了对柱状图和折线图的初始化和绘制,其中,使用 `Object.keys()` 方法遍历数据结构,拼装出需要的数据格式。最后,调用 `setOption()` 方法设置 echarts 的配置项和数据,绘制出图表。
相关推荐
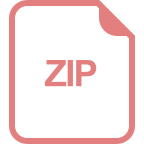
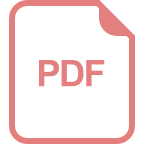













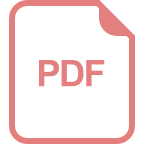