javagui界面设计
时间: 2023-07-21 08:38:57 浏览: 38
Java中的GUI界面设计通常使用Swing库。以下是一些设计GUI界面的基本步骤:
1. 导入Swing库。
```
import javax.swing.*;
```
2. 创建一个JFrame对象,它是一个顶级容器,用于包含和组织Swing组件。
```
JFrame frame = new JFrame("My Application");
```
3. 设置JFrame窗口大小和位置。
```
frame.setSize(400, 300);
frame.setLocationRelativeTo(null);
```
4. 创建Swing组件,例如JLabel、JButton、JTextField等。
```
JLabel label = new JLabel("Enter your name:");
JTextField textField = new JTextField(20);
JButton button = new JButton("Submit");
```
5. 将组件添加到JFrame窗口中。
```
frame.add(label);
frame.add(textField);
frame.add(button);
```
6. 设置组件的位置和大小。
```
label.setBounds(50, 50, 150, 30);
textField.setBounds(200, 50, 150, 30);
button.setBounds(150, 100, 100, 30);
```
7. 显示JFrame窗口。
```
frame.setVisible(true);
```
完整的示例代码如下:
```
import javax.swing.*;
public class MyApplication {
public static void main(String[] args) {
JFrame frame = new JFrame("My Application");
frame.setSize(400, 300);
frame.setLocationRelativeTo(null);
JLabel label = new JLabel("Enter your name:");
JTextField textField = new JTextField(20);
JButton button = new JButton("Submit");
frame.add(label);
frame.add(textField);
frame.add(button);
label.setBounds(50, 50, 150, 30);
textField.setBounds(200, 50, 150, 30);
button.setBounds(150, 100, 100, 30);
frame.setVisible(true);
}
}
```
在这个示例中,我们创建了一个名为"My Application"的JFrame窗口,添加了一个标签、一个文本框和一个按钮。然后我们设置了它们的位置和大小,并通过调用setVisible方法来显示JFrame窗口。
相关推荐
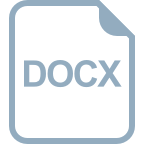








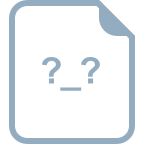
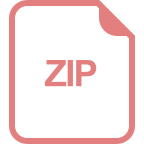
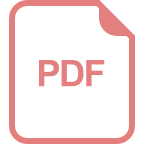
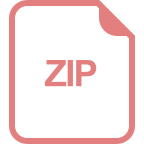
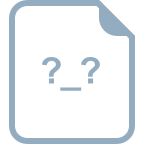