用opencv识别一张图片里面特定形状面积占比,用c++实现
时间: 2024-02-09 11:08:29 浏览: 23
好的,以下是使用 C++ 实现的代码示例:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图片
Mat img = imread("image.jpg");
// 将图片转换为灰度图
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
// 二值化处理
Mat thresh;
threshold(gray, thresh, 127, 255, THRESH_BINARY);
// 查找轮廓
vector<vector<Point>> contours;
vector<Vec4i> hierarchy;
findContours(thresh, contours, hierarchy, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 遍历轮廓
for (size_t i = 0; i < contours.size(); i++)
{
// 计算轮廓面积
double area = contourArea(contours[i]);
// 计算图片总面积
double total_area = img.rows * img.cols;
// 计算特定形状的面积占比
if (area / total_area > 0.5) // 假设特定形状的面积占比大于 50%
{
// 在原图上绘制轮廓
drawContours(img, contours, i, Scalar(0, 0, 255), 2);
}
}
// 显示结果
imshow("image", img);
waitKey(0);
destroyAllWindows();
return 0;
}
```
这段代码与 Python 版本的实现非常类似。我们首先读取一张图片,然后将其转换为灰度图并进行二值化处理。接着使用 `findContours()` 函数查找轮廓,然后遍历每个轮廓,计算其面积并与图片总面积比较,从而确定特定形状的面积占比是否达到要求。如果达到要求,则在原图上绘制该轮廓。
你可以根据自己的需要,修改代码中的阈值、特定形状的面积占比等参数,以达到更好的效果。
相关推荐
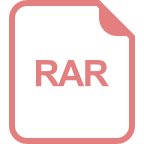
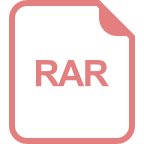
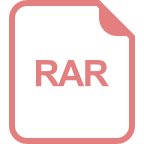














