getTheName: (ids) => { const namesArr = []; const idsArr = ids.split(","); table.members.map((i) => { idsArr.map((j) => { if (i.uid == j) { namesArr.push(i.nickname); } }); }); return namesArr; },报错Uncaught (in promise) TypeError: Cannot read properties of null (reading 'split'),如何修改
时间: 2024-04-14 15:26:35 浏览: 19
根据您提供的代码,错误是在执行 `ids.split(",")` 时出现的。这个错误指示 `ids` 是一个空值(null)或未定义(undefined),无法使用 `split` 方法进行拆分。
要修复这个问题,您可以在调用 `split` 方法之前添加一个条件检查来确保 `ids` 不为空。您可以使用如下代码示例:
```javascript
getTheName: (ids) => {
const namesArr = [];
if (ids) { // 添加条件检查
const idsArr = ids.split(",");
table.members.map((i) => {
idsArr.map((j) => {
if (i.uid == j) {
namesArr.push(i.nickname);
}
});
});
}
return namesArr;
}
```
通过添加条件检查,只有在 `ids` 不为空时才会执行拆分操作,从而避免了出现该错误。请注意,这只是一个示例修复,具体修复方法可能需要根据您的代码逻辑进行调整。
相关问题
import { onMounted, reactive } from "vue"; import { getPatrolReportList, getUserList ,deletePatrolList} from "@/views/Api/index.js";const table = reactive({ data: [], search: { username: "", search: "", }, members: [], //收费员集合 scopeId, columns: [ { label: "序号", type: "index" }, { label: "巡查员", prop: "nickname", width: 120 }, { label: "路段", prop: "parking_name", width: 200 }, { label: "罚金(元)", prop: "deduction", width: 200 }, { label: "时间", prop: "create_time", width: 180 }, { label: "原因", prop: "content" }, ], loading: false, paging: [1, 20], total: 0, height: "70vh", getColumns() { exportOption.columns = [...new Set(table.columns)]; exportOption.columns.shift(); }, getData: async (num) => { table.loading = true; if (typeof num == "number") table.paging[0] = num; const { data } = await getPatrolReportList({ paging: table.paging, ...table.search, }); table.total = data?.total; table.loading = false; table.data = data?.data; table.data.map((i) => { if (i.pics) { i.pics = i.pics.slice(1, i.pics.length - 1).split(","); i.members = table.getTheName(i.uids); } }); }, // 获取收费员 getMembers: async () => { const { data } = await getUserList({ paging: [1, 500], role_id: 19, }); table.members = data.data; table.getData(); }, // 获取惩罚数据中的收费员姓名 getTheName: (ids) => { const namesArr = []; if (ids) { const idsArr = ids.split(","); table.members.map((i) => { idsArr.map((j) => { if (i.uid == j) { namesArr.push(i.nickname); } }); }); } return namesArr; }, // 撤销惩罚 async del({ parking }) { const { error } = await deletePatrolList({ id: parking.map((m) => m.parking_patrol_id).join(","), }); if (error) return; $message.warning("已解绑!"); table.getData(); }, });这段代码报错Uncaught (in promise) TypeError: Cannot read properties of undefined (reading 'map')该怎么解决
根据您提供的代码,错误是在调用 `map` 方法时出现的,指示无法读取 undefined 的属性 'map'。
这个错误通常发生在尝试对未定义(undefined)的对象使用 `map` 方法时。在您的代码中,错误出现在 `del` 方法中的 `parking.map((m) => m.parking_patrol_id)` 这一行代码。
要解决这个问题,您可以添加条件检查来确保 `parking` 对象存在并且不为空。您可以使用如下代码示例:
```javascript
async del({ parking }) {
if (parking && Array.isArray(parking)) { // 添加条件检查
const { error } = await deletePatrolList({
id: parking.map((m) => m.parking_patrol_id).join(","),
});
if (error) return;
$message.warning("已解绑!");
table.getData();
}
}
```
通过添加条件检查,只有在 `parking` 存在且为数组时才会执行 `map` 方法,从而避免了出现该错误。
请注意,以上是一种常见的修复方法,具体修复方法可能需要根据您的代码逻辑和需求进行调整。如果问题仍然存在或您需要更多帮助,请提供更多相关的代码或上下文信息。
const _ids = row.id || ids.value;
const _ids = row.id || ids.value; 这段代码是一个变量赋值语句,它的作用是将变量_ids赋值为row.id的值,如果row.id的值为假(例如undefined、null、false等),则将ids.value的值赋给_ids。
在这段代码中,使用了逻辑或运算符(||)。逻辑或运算符的规则是,如果第一个操作数为真,则返回第一个操作数的值;如果第一个操作数为假,则返回第二个操作数的值。
如果row.id的值为真,则_ids的值将为row.id的值;如果row.id的值为假,则_ids的值将为ids.value的值。
相关推荐
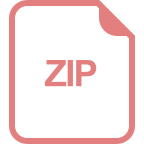
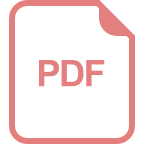
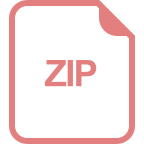













