请用C#语言,写一段完整代码,实现CAD拾取点填充的功能
时间: 2024-02-25 11:51:53 浏览: 137
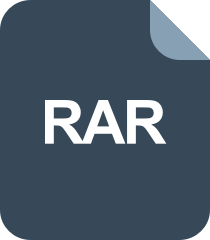
C#实现CAD原代码

以下是一个使用C#语言实现CAD拾取点填充的示例代码。请注意,这只是一个示例,需要根据实际需要进行修改和调整。
```csharp
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.EditorInput;
using Autodesk.AutoCAD.Geometry;
using Autodesk.AutoCAD.Runtime;
namespace MyCommands
{
public class MyCommandsClass
{
[CommandMethod("FILL")]
public void FillCommand()
{
// 获取当前文档和编辑器对象
Document doc = Application.DocumentManager.MdiActiveDocument;
Editor ed = doc.Editor;
// 提示用户选择要填充的区域
PromptEntityOptions peo = new PromptEntityOptions("\n选择要填充的区域: ");
peo.SetRejectMessage("\n请选择一个封闭的区域.");
peo.AddAllowedClass(typeof(Polyline), true);
peo.AddAllowedClass(typeof(Circle), true);
peo.AddAllowedClass(typeof(Arc), true);
peo.AllowNone = false;
PromptEntityResult per = ed.GetEntity(peo);
if (per.Status != PromptStatus.OK)
return;
// 获取实体对象
Entity ent = (Entity)doc.TransactionManager.GetObject(per.ObjectId, OpenMode.ForWrite);
// 判断实体是否是封闭的
if (!ent.Closed)
{
ed.WriteMessage("\n选择的实体不是封闭的,请重新选择.");
return;
}
// 提示用户选择填充样式
PromptKeywordOptions pko = new PromptKeywordOptions("\n选择填充样式: ");
pko.Keywords.Add("SOLID");
pko.Keywords.Add("HATCH");
pko.AllowNone = false;
PromptResult pkr = ed.GetKeywords(pko);
if (pkr.Status != PromptStatus.OK)
return;
// 创建填充对象
using (Transaction tr = doc.TransactionManager.StartTransaction())
{
BlockTableRecord btr = (BlockTableRecord)doc.Database.CurrentSpaceId.GetObject(OpenMode.ForWrite);
Hatch hatch = new Hatch();
hatch.SetDatabaseDefaults();
if (pkr.StringResult == "SOLID")
{
hatch.PatternScale = 1;
hatch.PatternAngle = 0;
hatch.PatternType = HatchPatternType.PreDefined;
hatch.SetHatchPattern(HatchPatternType.PreDefined, "SOLID");
}
else if (pkr.StringResult == "HATCH")
{
// TODO: 选择填充图案和颜色
}
btr.AppendEntity(hatch);
tr.AddNewlyCreatedDBObject(hatch, true);
// 添加选择的区域
hatch.AppendLoop(HatchLoopTypes.Default, new ObjectIdCollection() { ent.ObjectId });
// 设置填充的属性
hatch.EvaluateHatch(true);
hatch.ColorIndex = 1;
hatch.Layer = "0";
tr.Commit();
}
ed.WriteMessage("\n填充完成.");
}
}
}
```
阅读全文
相关推荐
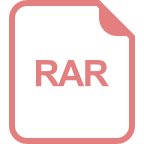
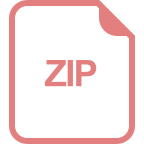
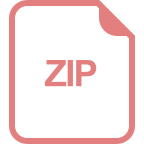
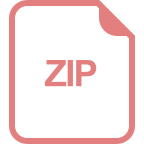
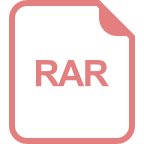
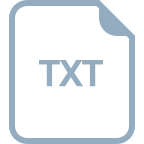
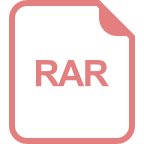
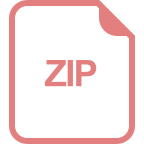
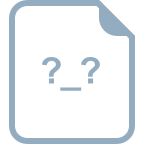
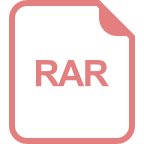
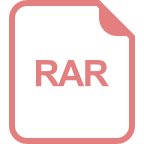
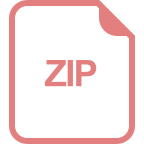