编译原理词法分析器C语言版
时间: 2023-07-07 19:19:00 浏览: 118
好的,我可以为您解答关于编译原理词法分析器的问题。首先,词法分析器是编译器中的一个重要组成部分,它的作用是将源代码转换为词法单元(Token)序列。在C语言中,我们可以使用有限自动机(DFA)来实现词法分析器。
以下是一个简单的C语言版词法分析器示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
#define MAX_LENGTH 100
typedef enum {
ID,
NUM,
PLUS,
MINUS,
TIMES,
DIVIDE,
ASSIGN,
SEMI,
LPAREN,
RPAREN,
ERROR
} TokenType;
typedef struct {
TokenType type;
char value[MAX_LENGTH];
} Token;
Token scan() {
Token token;
char ch = getchar();
while (isspace(ch)) {
ch = getchar();
}
if (isalpha(ch)) {
int i = 0;
while (isalnum(ch)) {
token.value[i++] = ch;
ch = getchar();
}
token.value[i] = '\0';
ungetc(ch, stdin);
token.type = ID;
} else if (isdigit(ch)) {
int i = 0;
while (isdigit(ch)) {
token.value[i++] = ch;
ch = getchar();
}
token.value[i] = '\0';
ungetc(ch, stdin);
token.type = NUM;
} else if (ch == '+') {
token.type = PLUS;
} else if (ch == '-') {
token.type = MINUS;
} else if (ch == '*') {
token.type = TIMES;
} else if (ch == '/') {
token.type = DIVIDE;
} else if (ch == '=') {
token.type = ASSIGN;
} else if (ch == ';') {
token.type = SEMI;
} else if (ch == '(') {
token.type = LPAREN;
} else if (ch == ')') {
token.type = RPAREN;
} else {
token.type = ERROR;
}
return token;
}
int main() {
Token token;
while ((token = scan()).type != ERROR) {
printf("%s: %s\n", token.value, token.type);
}
return 0;
}
```
在这个例子中,我们定义了一个`Token`结构体,包含了`Token`的类型和值。然后通过`scan()`函数实现了词法分析,根据不同的字符类型返回不同的`Token`。最后在`main()`函数中,循环调用`scan()`函数,输出每个`Token`的类型和值。
需要注意的是,这个例子只是一个简单的示例,实际上词法分析器的实现要更加复杂,需要考虑到关键字、运算符、注释等情况。不过通过这个例子,您可以了解到词法分析器的基本原理,以及如何使用C语言实现。
阅读全文
相关推荐
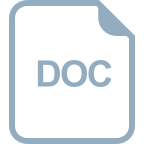
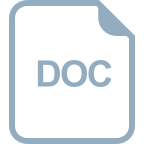
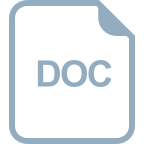
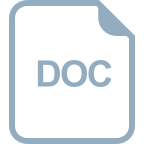
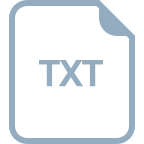
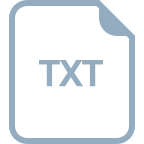
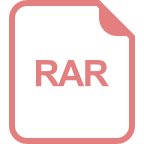
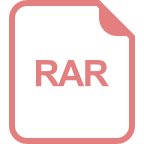
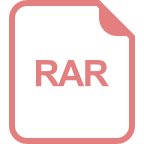
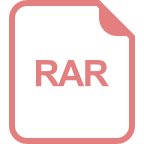
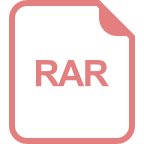
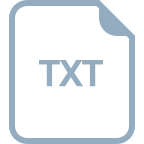
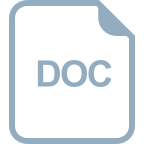
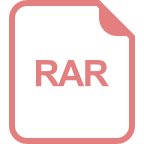
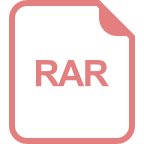