请用Vue框架编写以下完整需求1: 课程编号不需要输入,是自动生成的,规则:最后一个门课程编号+1。如果删除所有的 课程,编号从2023001开始。 需求2: 课程信息显示时,数组中是没有序列,需要再循环显示的时候做处理。 需求3: 学时大于25的为必修课,小于等于25为选修课。 需求4: 实现删除和清除数据。 删除的提示,删除空数据的提示。不能添加空的课程信息。
时间: 2024-03-06 12:49:20 浏览: 73
好的,以下是使用Vue框架实现的完整代码:
```
<template>
<div>
<h2>课程管理</h2>
<form>
<label for="name">课程名称:</label>
<input type="text" id="name" v-model="course.name" required/><br/>
<label for="teacher">授课教师:</label>
<input type="text" id="teacher" v-model="course.teacher" required/><br/>
<label for="hours">学时:</label>
<input type="number" id="hours" v-model="course.hours" required/><br/>
<button type="button" @click="addCourse">添加课程</button>
<button type="button" @click="clearData">清除数据</button>
</form>
<h2>课程列表</h2>
<table>
<thead>
<tr>
<th>课程编号</th>
<th>课程名称</th>
<th>授课教师</th>
<th>学时</th>
<th>是否必修</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(course, index) in sortedCourses" :key="course.id">
<td>{{ course.id }}</td>
<td>{{ course.name }}</td>
<td>{{ course.teacher }}</td>
<td>{{ course.hours }}</td>
<td>{{ course.hours > 25 ? "必修" : "选修" }}</td>
<td>
<button type="button" @click="deleteCourse(index)">删除</button>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
courses: [
{
id: 2023001,
name: "Vue框架入门",
teacher: "张三",
hours: 30
}
],
course: {
name: "",
teacher: "",
hours: null
}
};
},
methods: {
addCourse() {
if (!this.course.name || !this.course.teacher || !this.course.hours) {
alert("请填写完整的课程信息!");
return;
}
const lastId = this.courses.length > 0 ? this.courses[this.courses.length - 1].id : 2023000;
const newCourse = {
id: lastId + 1,
name: this.course.name,
teacher: this.course.teacher,
hours: this.course.hours
};
this.courses.push(newCourse);
this.course.name = "";
this.course.teacher = "";
this.course.hours = null;
},
deleteCourse(index) {
if (confirm("确定要删除这门课程吗?")) {
this.courses.splice(index, 1);
alert("删除成功!");
}
},
clearData() {
if (confirm("确定要清空所有课程吗?")) {
this.courses = [];
alert("清空成功!");
}
}
},
computed: {
sortedCourses() {
return this.courses.sort((a, b) => a.id - b.id);
}
}
};
</script>
```
具体说明:
1. 需求1:通过 `lastId` 计算出自动生成的课程编号,并在添加课程时将其加入课程数组 `courses` 中。
2. 需求2:通过 `sortedCourses` 计算出按课程编号排序后的课程数组,并在循环显示时使用。
3. 需求3:通过判断 `course.hours` 的大小,计算出课程是否为必修课。
4. 需求4:通过 `deleteCourse` 和 `clearData` 方法实现删除和清除数据的功能,并在删除前进行提示和空数据判断。
阅读全文
相关推荐
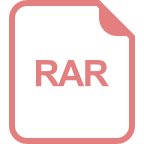
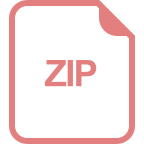
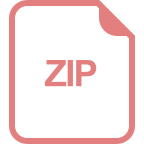

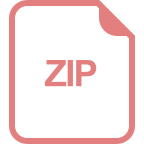
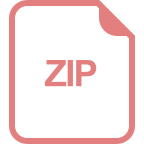
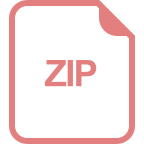
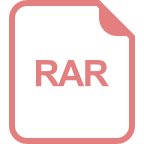
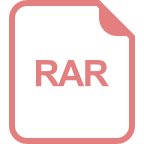
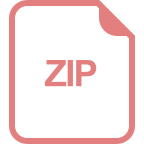
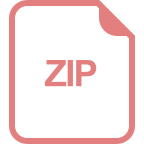
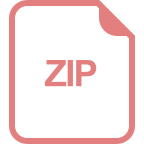
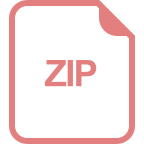
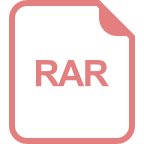
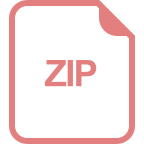
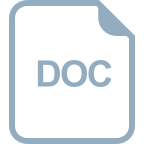
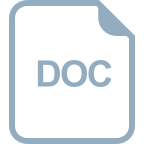
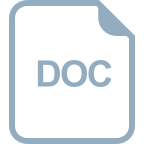
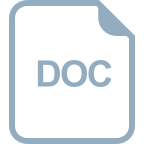
