用stm32f103c8t6的pc14作为ds18b20温度传感器DQ引脚,写一段测量温度的完整代码包括头文件
时间: 2023-11-01 11:06:40 浏览: 33
很高兴你使用ChitGPT进行对话,我会尽力提供帮助。以下是使用stm32f103c8t6的代码来测量ds18b20温度传感器的温度:
```c
#include <stdio.h>
#include "stm32f10x.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_rcc.h"
#include "delay.h"
#define GPIO_DS18B20 GPIOC
#define PIN_DS18B20 GPIO_Pin_14
void ds18b20_init() {
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_OD; // 设置输出口开漏输出
GPIO_InitStruct.GPIO_Pin = PIN_DS18B20;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIO_DS18B20, &GPIO_InitStruct);
GPIO_SetBits(GPIO_DS18B20, PIN_DS18B20); // DQ引脚为高电平
delay_us(500);
}
uint8_t ds18b20_reset(void) {
// DQ 引脚拉低
GPIO_ResetBits(GPIO_DS18B20, PIN_DS18B20);
delay_us(750);
// DQ 引脚拉高
GPIO_SetBits(GPIO_DS18B20, PIN_DS18B20);
delay_us(15);
// 读取 DQ 引脚的电平
uint8_t q = GPIO_ReadInputDataBit(GPIO_DS18B20, PIN_DS18B20);
delay_us(220);
// 返回 DQ 引脚的状态
return q;
}
void ds18b20_write_bit(uint8_t bit) {
// DQ 引脚拉低
GPIO_ResetBits(GPIO_DS18B20, PIN_DS18B20);
delay_us(2);
if (bit) {
// DQ 引脚拉高
GPIO_SetBits(GPIO_DS18B20, PIN_DS18B20);
}
delay_us(60);
// DQ 引脚拉高
GPIO_SetBits(GPIO_DS18B20, PIN_DS18B20);
delay_us(2);
}
uint8_t ds18b20_read_bit(void) {
// DQ 引脚拉低
GPIO_ResetBits(GPIO_DS18B20, PIN_DS18B20);
delay_us(2);
// DQ 引脚拉高
GPIO_SetBits(GPIO_DS18B20, PIN_DS18B20);
delay_us(10);
// 读取 DQ 引脚的状态
uint8_t q = GPIO_ReadInputDataBit(GPIO_DS18B20, PIN_DS18B20);
delay_us(48);
// 返回 DQ 引脚的状态
return q;
}
void ds18b20_write_byte(uint8_t byte) {
for (int i = 0; i < 8; i++) {
ds18b20_write_bit(byte & 0x01);
byte >>= 1;
}
}
uint8_t ds18b20_read_byte(void) {
uint8_t res = 0;
for (int i = 0; i < 8; i++) {
res >>= 1;
if (ds18b20_read_bit()) {
res |= 0x80;
}
}
return res;
}
float ds18b20_read_temperature(void) {
float temp = 0.0;
if (ds18b20_reset() == 0) {
ds18b20_write_byte(0xcc); // 跳过 ROM
ds18b20_write_byte(0x44); // 启动温度转换
delay_ms(800); // 等待转换完成
ds18b20_reset(); // 从写模式切换到读模式
ds18b20_write_byte(0xcc); // 跳过 ROM
ds18b20_write_byte(0xbe); // 读取温度值
uint16_t temp_raw = ds18b20_read_byte();
temp_raw |= ds18b20_read_byte() << 8;
temp = (float) temp_raw / 16;
}
return temp;
}
int main(void) {
ds18b20_init();
float temperature = ds18b20_read_temperature();
printf("The temperature is %.2f degrees Celsius.\n", temperature);
return 0;
}
```
在这个代码中,DQ引脚的控制使用了 GPIOC14,使用了开漏输出以及时间延迟函数来控制数据的传输。代码中包含了 ds18b20 初始化、重置、写入位和字节,读取位和字节和读取温度等功能函数。
相关推荐
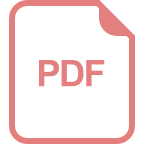
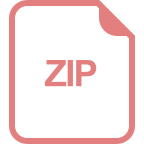
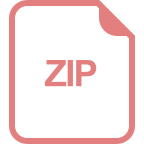














