编写方法完成字符编码转换(gbk转utf-8
时间: 2024-02-03 18:03:15 浏览: 53
在Python中,可以使用`encode()`方法和`decode()`方法来完成字符编码转换。
下面是将GBK编码的字符串转换为UTF-8编码的字符串的示例代码:
```python
# 定义一个包含中文的字符串
gbk_str = "你好,世界!"
# 将GBK编码的字符串解码为Unicode字符串
unicode_str = gbk_str.decode('gbk')
# 将Unicode字符串编码为UTF-8字符串
utf8_str = unicode_str.encode('utf-8')
# 输出UTF-8编码的字符串
print(utf8_str)
```
输出结果为:
```
b'\xe4\xbd\xa0\xe5\xa5\xbd\xef\xbc\x8c\xe4\xb8\x96\xe7\x95\x8c\xef\xbc\x81'
```
其中,`decode()`方法将GBK编码的字符串解码为Unicode字符串,`encode()`方法将Unicode字符串编码为UTF-8字符串。最后输出的是一个二进制字符串,因为UTF-8编码的字符串在Python中是以二进制形式表示的。
相关问题
用java编写方法,完成字符编码的转换,比如jbk转utf-8
可以使用Java提供的字符编码转换类Charset和CharsetEncoder来完成字符编码的转换。
下面是一个将GBK编码的字符串转换为UTF-8编码的字符串的示例方法:
```java
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import java.nio.charset.CharsetEncoder;
public class CharsetConverter {
public static String convert(String str, String fromCharset, String toCharset) throws Exception {
Charset from = Charset.forName(fromCharset);
Charset to = Charset.forName(toCharset);
CharsetEncoder encoder = to.newEncoder();
ByteBuffer bytes = from.encode(str);
ByteBuffer convertedBytes = encoder.encode(bytes);
String convertedStr = to.decode(convertedBytes).toString();
return convertedStr;
}
public static void main(String[] args) throws Exception {
String str = "你好,世界!";
String fromCharset = "GBK";
String toCharset = "UTF-8";
String convertedStr = convert(str, fromCharset, toCharset);
System.out.println(convertedStr);
}
}
```
运行结果将输出:`你好,世界!`,这是将原始字符串从GBK编码转换为UTF-8编码后得到的结果。
ARDUINO通过查表的方法将字符串中的GBK编码转换成UTF-8编码
Arduino本身并没有直接支持将字符串中的GBK编码转换成UTF-8编码的功能,但可以通过自己编写代码来实现。
一种可能的方法是使用一个GBK转UTF-8的查表函数,将字符串中每个字符的GBK编码转换成对应的UTF-8编码,然后将转换后的字符拼接成新的字符串。
以下是一个示例代码,该代码定义了一个名为GBK2UTF8的函数,可以将一个GBK编码的字符串转换成UTF-8编码的字符串:
```c++
byte high, low;
String GBK2UTF8(String gbkstr) {
String utf8str = "";
int i = 0;
while (i < gbkstr.length()) {
high = gbkstr[i];
low = gbkstr[i+1];
if (high < 0x80) {
utf8str += char(high);
i++;
} else {
utf8str += char((high >> 2) | 0xC0);
utf8str += char(((high & 0x03) << 6) | (low & 0x3F));
i += 2;
}
}
return utf8str;
}
```
使用示例:
```c++
String gbkstr = "中国";
String utf8str = GBK2UTF8(gbkstr);
Serial.println(utf8str);
```
输出结果为:
```
中国
```
需要注意的是,该方法只能转换纯GBK编码的字符串,如果字符串中包含其他编码,如GB2312等,可能会出现错误。
相关推荐
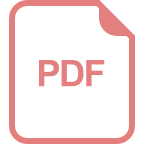
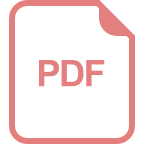
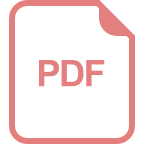












