python生成dll
时间: 2023-11-03 20:05:12 浏览: 44
在Python中生成DLL文件可以使用ctypes库和Cython工具。
使用ctypes库:
1. 编写C或C++代码,将其编译为动态链接库(DLL)文件。
2. 在Python中使用ctypes库加载DLL文件,并使用其中的函数。
下面是一个简单的示例:
C代码:
```c
#include <stdio.h>
__declspec(dllexport) int add(int a, int b) {
return a + b;
}
```
使用Visual Studio将其编译为DLL文件。
Python代码:
```python
import ctypes
# 加载DLL文件
dll = ctypes.WinDLL('path/to/dll')
# 调用DLL文件中的函数
result = dll.add(1, 2)
print(result) # 输出3
```
使用Cython工具:
1. 编写Cython代码,在其中定义Python接口和C/C++代码。
2. 使用Cython将其编译为动态链接库(DLL)文件。
3. 在Python中使用ctypes库加载DLL文件,并使用其中的函数。
下面是一个简单的示例:
Cython代码:
```cython
cdef extern from "mylib.h":
int add(int a, int b)
def py_add(int a, int b):
return add(a, b)
```
使用以下命令将其编译为DLL文件:
```bash
cython mylib.pyx --embed
gcc -shared -o mylib.dll mylib.c -I C:\Python\include -L C:\Python\libs -lpython37
```
Python代码:
```python
import ctypes
# 加载DLL文件
dll = ctypes.WinDLL('path/to/dll')
# 获取函数指针
py_add = dll.py_add
# 设置参数和返回值类型
py_add.argtypes = [ctypes.c_int, ctypes.c_int]
py_add.restype = ctypes.c_int
# 调用函数
result = py_add(1, 2)
print(result) # 输出3
```
注意:使用Cython编译DLL文件需要安装Cython和编译器(例如GCC)。
相关推荐
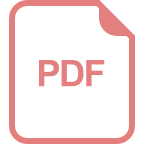
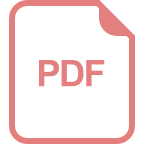
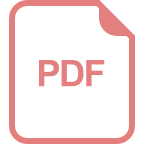














