如何在C++中有一个sstring类型的username,现创建指针指向mysql中的表将表和username连接起来,可以在C++中对mysql中的表进行增删查改的操作
时间: 2024-02-22 07:55:56 浏览: 51
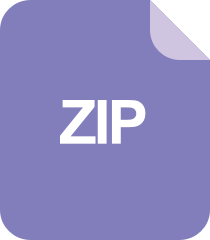
通过API函数对Mysql数据库实现增、删、改、查操作C++实现
要在C++中连接到MySQL数据库,您需要使用MySQL C++ Connector API。以下是实现该功能的基本步骤:
1. 安装MySQL C++ Connector API,并在代码中包含相关的头文件。
2. 建立与数据库的连接,您需要提供主机名、用户名、密码和数据库名称等信息。
3. 执行SQL查询,您可以使用MySQL C++ Connector API提供的函数将查询发送到MySQL服务器。
4. 处理SQL查询结果,您可以使用MySQL C++ Connector API提供的函数获取查询结果,并将其存储在您的C++变量中。
以下是一个简单的示例程序,该程序使用MySQL C++ Connector API连接到数据库并执行查询:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <mysql_driver.h>
#include <mysql_connection.h>
#include <mysql_error.h>
#include <cppconn/statement.h>
#include <cppconn/resultset.h>
using namespace std;
using namespace sql;
int main()
{
// 建立与数据库的连接
const string host = "localhost";
const string user = "root";
const string password = "password";
const string database = "test";
unique_ptr<Driver> driver(new mysql::Driver);
unique_ptr<Connection> conn(driver->connect(host, user, password));
conn->setSchema(database);
// 执行SQL查询
string username = "example";
stringstream ss;
ss << "SELECT * FROM users WHERE username = '" << username << "'";
string query = ss.str();
unique_ptr<Statement> stmt(conn->createStatement());
unique_ptr<ResultSet> res(stmt->executeQuery(query));
// 处理SQL查询结果
while (res->next()) {
string id = res->getString("id");
string name = res->getString("name");
string email = res->getString("email");
cout << "id: " << id << ", name: " << name << ", email: " << email << endl;
}
return 0;
}
```
在上面的示例中,我们使用stringstream将变量username插入到SQL查询中。我们还使用ResultSet对象处理查询结果,并输出每行的id、name和email。
注意,在实际应用中,您需要对SQL查询进行参数化处理,以防止SQL注入攻击。此外,您需要处理连接和查询错误,以确保程序在出现错误时能够正常终止。
阅读全文
相关推荐
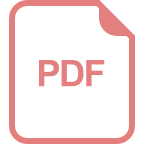
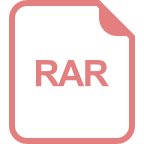
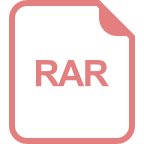
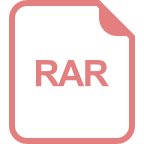
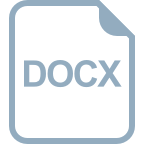
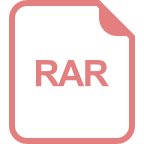
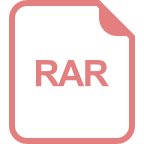
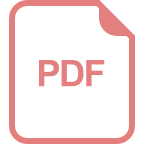
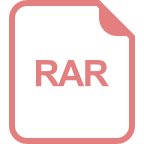
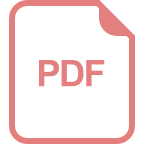
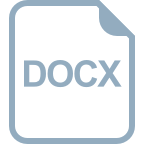
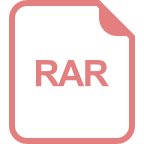
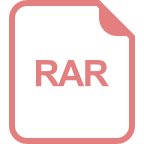
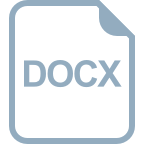
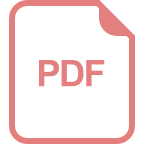
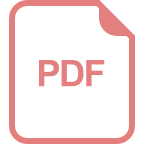