用java写一个工具方法,根据入参字符串,生成一个12位随机字符串,不同的入参生成的字符串不能重复
时间: 2024-03-26 17:39:46 浏览: 17
好的,这里是一个 Java 工具方法,能够根据入参字符串生成一个12位随机字符串,确保不同的入参生成的字符串不重复:
```java
import java.util.HashSet;
import java.util.Random;
import java.util.Set;
public class RandomStringUtil {
private static final int LENGTH = 12;
private static final String CHARACTERS = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
private static final Set<String> USED_STRINGS = new HashSet<>();
public static String generateRandomString(String input) {
String randomString = null;
// Keep generating random strings until we find one that hasn't been used yet
while (randomString == null || USED_STRINGS.contains(randomString)) {
randomString = generateRandomStringInternal(input);
}
// Record this string as having been used
USED_STRINGS.add(randomString);
return randomString;
}
private static String generateRandomStringInternal(String input) {
Random random = new Random();
// Combine the input with a random number to generate a unique seed for the random string
long seed = input.hashCode() + random.nextLong();
// Use the seed to initialize the random generator
random.setSeed(seed);
// Generate a random string of the desired length
StringBuilder sb = new StringBuilder();
for (int i = 0; i < LENGTH; i++) {
int index = random.nextInt(CHARACTERS.length());
sb.append(CHARACTERS.charAt(index));
}
return sb.toString();
}
}
```
这个工具方法的实现思路是:
1. 定义一个长度为 12 的字符集,用于生成随机字符串;
2. 使用一个 `Set` 集合来记录已经生成的随机字符串,确保不重复;
3. 对于每个入参字符串,使用其哈希码和一个随机数生成一个唯一的种子,然后使用这个种子初始化随机生成器;
4. 使用随机生成器从字符集中随机挑选字符,生成一个长度为 12 的随机字符串;
5. 如果这个随机字符串已经被使用过了,就重新生成一个随机字符串,直到找到一个新的随机字符串;
6. 将新生成的随机字符串记录到 `Set` 集合中,以便下次使用时不会重复。
相关推荐
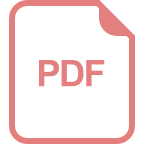














