代码优化 def process_data(data): # 处理数据逻辑 print("Processing data:", data) def push_data(data_queue:queue.Queue): while True: data_queue.put(str("123")) time.sleep(0.5) # Press the green button in the gutter to run the script. if __name__ == '__main__': print_hi('PyCharm') data_queue = queue.Queue() data_thread = threading.Thread(target=process_data, args=(data_queue,)) data_thread.start() # 创建线程池 executor = ThreadPoolExecutor(max_workers=5) # 从队列中获取数据并处理 while True: data = data_queue.get() if data: print('data = {}'.format(data)) # 提交任务到线程池 executor.submit(process_data, data)
时间: 2024-02-25 12:59:47 浏览: 94
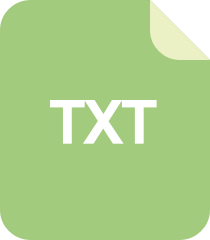
数据处理部分代码,看一下还能优化吗?
在这段代码中,我们可以看到有一些可以进行优化的部分。
首先,`process_data`函数中的处理逻辑看起来很简单,可以直接在`push_data`函数中完成,避免创建多个线程来处理。因此,我们可以将`process_data`函数的内容移动到`push_data`函数中,然后将`process_data`函数从`ThreadPoolExecutor`中删除,直接在`executor.submit`中调用`push_data`函数即可。
其次,`push_data`函数中的无限循环可以使用`while`循环和`Event`对象来实现,避免在循环中使用`time.sleep`来等待一段时间。这样可以使程序更加高效和可控。
最后,我们可以使用`with`语句来自动管理线程池,避免手动关闭线程池时出现问题。
下面是优化后的代码:
```python
import queue
import threading
from concurrent.futures import ThreadPoolExecutor, as_completed
from threading import Event
def push_data(data_queue: queue.Queue):
while not stop_event.is_set():
data = "123"
data_queue.put(data)
stop_event.wait(0.5)
def process_data(data):
# 处理数据逻辑
print("Processing data:", data)
if __name__ == '__main__':
data_queue = queue.Queue()
stop_event = Event()
# 创建线程池
with ThreadPoolExecutor(max_workers=5) as executor:
# 启动数据生产线程
t = threading.Thread(target=push_data, args=(data_queue,))
t.start()
# 从队列中获取数据并处理
while not stop_event.is_set():
try:
data = data_queue.get(timeout=1)
except queue.Empty:
continue
# 提交任务到线程池
future = executor.submit(process_data, data)
future.add_done_callback(lambda f: f.result())
# 关闭数据生产线程
stop_event.set()
t.join()
```
在优化后的代码中,我们使用`while`循环和`Event`对象来实现线程的控制。`push_data`函数中的循环会在`stop_event`对象被设置时结束,而不需要使用`time.sleep`等待一段时间。同时,我们使用`with`语句来自动管理线程池,避免手动关闭线程池时出现问题。
阅读全文
相关推荐
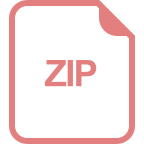
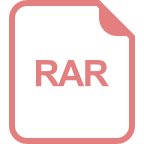

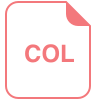
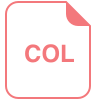
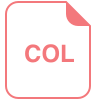
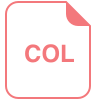
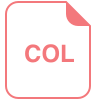
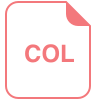
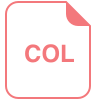
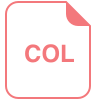
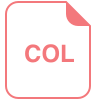
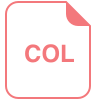
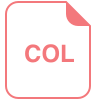
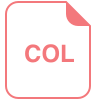
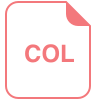
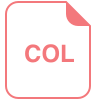
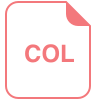