编写一个获取cookie的代码
时间: 2024-04-30 11:22:24 浏览: 68
以下是一个获取cookie的示例代码:
```python
import requests
# 定义请求头,包含 User-Agent 和 Referer
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3',
'Referer': 'https://www.example.com/'
}
# 发送 GET 请求
response = requests.get('https://www.example.com/', headers=headers)
# 获取响应中的 cookie
cookie = response.cookies.get_dict()
# 打印 cookie
print(cookie)
```
在上面的代码中,我们使用 `requests` 发送了一个 GET 请求,并在请求头中添加了 `User-Agent` 和 `Referer`。然后我们通过 `response.cookies.get_dict()` 方法获取了响应中的 cookie,并将其打印出来。
相关问题
有一个用户名 test, 密码 12345, 请编写一段创建COOKIE的JSP代码,将用户名和密码保存到COOKIE中, 然后再编写一段读取COOKIE的JSP代码, 在页面上将COOKIE的信息显示出来。
好的,以下是创建和读取 COOKIE 的 JSP 代码示例:
创建 COOKIE 的 JSP 代码:
```
<%
String username = "test";
String password = "12345";
// 创建名为 user 的 COOKIE,值为 username 和 password
Cookie userCookie = new Cookie("user", username + "&" + password);
// 设置 COOKIE 有效期为 24 小时
userCookie.setMaxAge(24 * 60 * 60);
// 将 COOKIE 添加到响应中
response.addCookie(userCookie);
%>
```
上述代码中,将用户名和密码拼接成一个字符串并保存到名为 "user" 的 COOKIE 中,设置 COOKIE 的有效期为 24 小时,并将 COOKIE 添加到响应中。
读取 COOKIE 的 JSP 代码:
```
<%
Cookie[] cookies = request.getCookies();
String username = null;
String password = null;
// 遍历所有的 COOKIE,查找名为 user 的 COOKIE
if (cookies != null) {
for (Cookie cookie : cookies) {
if (cookie.getName().equals("user")) {
String[] userValues = cookie.getValue().split("&");
username = userValues[0];
password = userValues[1];
}
}
}
// 输出用户名和密码
out.print("用户名:" + username + "<br>");
out.print("密码:" + password);
%>
```
上述代码中,首先获取所有 COOKIE,然后遍历查找名为 "user" 的 COOKIE,并从中取出保存的用户名和密码,最后在页面上输出用户名和密码。
希望这个示例对你有所帮助!
请编写一段持久cookie代码
以下是使用 Node.js 和 Express 框架实现持久 Cookie 的代码示例:
```javascript
const express = require('express');
const app = express();
// 设置持久 Cookie
app.get('/set-cookie', (req, res) => {
res.cookie('username', 'John Doe', { maxAge: 30 * 24 * 60 * 60 * 1000 }); // Cookie 过期时间为 30 天
res.send('Cookie 已设置');
});
// 获取持久 Cookie
app.get('/get-cookie', (req, res) => {
const username = req.cookies.username;
if (username) {
res.send(`欢迎回来,${username}`);
} else {
res.send('您还未登录');
}
});
app.listen(3000, () => {
console.log('应用已启动');
});
```
在上面的示例中,我们通过 `res.cookie()` 方法设置了一个名为 `username` 的 Cookie,它的过期时间为 30 天。在接下来的请求中,我们可以通过 `req.cookies.username` 来获取该 Cookie 的值。这样,我们就可以实现持久 Cookie 功能了。
阅读全文
相关推荐

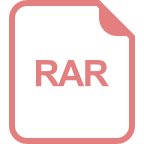











