if (strcmp(name, "varslot") == 0) { tv->tm_func = TmThreadsSlotVar; } else if (strcmp(name, "pktacqloop") == 0) { tv->tm_func = TmThreadsSlotPktAcqLoop; } else if (strcmp(name, "management") == 0) { tv->tm_func = TmThreadsManagement; } else if (strcmp(name, "command") == 0) { tv->tm_func = TmThreadsManagement; } else if (strcmp(name, "custom") == 0) { if (fn_p == NULL) goto error; tv->tm_func = fn_p; } else { printf("Error: Slot \"%s\" not supported\n", name); goto error; }
时间: 2023-12-29 14:02:35 浏览: 40
这段代码是一个条件语句,用于根据输入的字符串 name 来选择相应的函数指针赋值给 tv->tm_func 变量。具体来说,如果 name 是 "varslot",则赋值 TmThreadsSlotVar 函数指针;如果是 "pktacqloop",则赋值 TmThreadsSlotPktAcqLoop 函数指针;如果是 "management" 或 "command",则赋值 TmThreadsManagement 函数指针;如果是 "custom",则需要额外提供一个函数指针 fn_p,否则会跳转到 error 标签。如果 name 不属于以上任何一种情况,则会输出错误信息并跳转到 error 标签。
相关问题
include "head.h" int main(int argc, char *argv[]) { if (argc != 2) { printf("%s<dirname>\n", argv[0]); return -1; } struct dirent *dt; struct stat st; struct tm *tp; struct passwd *pd; struct group *gd; char pathname[300]; DIR *dir = opendir(argv[1]); while((dt= readdir(dir))!=NULL) { if (!strcmp(dt->d_name, ".") || !strcmp(dt->d_name, "..")) { continue; } memset(&stat, 0, sizeof(stat)); snprintf(pathname, strlen(argv[1]) + strlen(dt->d_name) + 2, "%s%s", argv[1], dt->d_name); if (lstat(pathname, &st) < 0) { perror("lstat"); return -1; } // 权限 if (S_ISREG(st.st_mode)) printf("-"); else if (S_ISDIR(st.st_mode)) printf("d"); else if (S_ISCHR(st.st_mode)) printf("c"); else if (S_ISBLK(st.st_mode)) printf("b"); else if (S_ISFIFO(st.st_mode)) printf("p"); else if (S_ISLNK(st.st_mode)) printf("l"); else if (S_ISSOCK(st.st_mode)) printf("s"); printf("111111111111111111111111\n"); int i; char *m = "xwr"; for (i = 8; i >= 0; i--) { if (st.st_mode & 1 << i) { printf("%c", m[i % 3]); } else { printf("-"); } } printf(" "); tp = localtime(&st.st_ctime); printf("%lu %s %s %ld", st.st_nlink, pd->pw_name, gd->gr_name, st.st_size); printf(" "); printf("%d月 ", tp->tm_mon + 1); printf("%d ", tp->tm_mday); printf("%d:%d ", tp->tm_hour, tp->tm_min); printf("%s ", pathname); } closedir(dir); return 0; }这段代码那里有问题
这段代码有一个问题是在使用 memset 函数清空结构体 st 时,传入了错误的参数。应该传入 &st 而不是 stat。正确的代码应该是:
```
memset(&st, 0, sizeof(st));
```
另外,pd 和 gd 指针变量在代码中没有进行初始化,可能会导致未定义的行为。应该使用 getpwuid 和 getgrgid 函数获取对应的用户和组信息。
#include "head.h" int main(int argc, char *argv[]) { if (argc != 2) { printf("%s<dirname>\n", argv[0]); return -1; } struct dirent *dt; struct stat st; struct tm *tp; struct passwd *pd; struct group *gd; char pathname[300]; DIR *dir = opendir(argv[1]); while((dt= readdir(dir))!=NULL) { if (!strcmp(dt->d_name, ".") || !strcmp(dt->d_name, "..")) { continue; } memset(&pd, 0, sizeof(pd)); memset(&st, 0, sizeof(st)); memset(&gd, 0, sizeof(gd)); snprintf(pathname, strlen(argv[1]) + strlen(dt->d_name) + 2, "%s%s", argv[1], dt->d_name); if (lstat(pathname, &st) < 0) { perror("lstat"); return -1; } // 权限 if (S_ISREG(st.st_mode)) printf("-"); else if (S_ISDIR(st.st_mode)) printf("d"); else if (S_ISCHR(st.st_mode)) printf("c"); else if (S_ISBLK(st.st_mode)) printf("b"); else if (S_ISFIFO(st.st_mode)) printf("p"); else if (S_ISLNK(st.st_mode)) printf("l"); else if (S_ISSOCK(st.st_mode)) printf("s"); int i; char *m = "xwr"; for (i = 8; i >= 0; i--) { if (st.st_mode & 1 << i) { printf("%c", m[i % 3]); } else { printf("-"); } } printf(" "); tp = localtime(&st.st_ctime); printf("%lu %s %s %ld", st.st_nlink, pd->pw_name, gd->gr_name, st.st_size); printf(" "); printf("%d月 ", tp->tm_mon + 1); printf("%d ", tp->tm_mday); printf("%d:%d ", tp->tm_hour, tp->tm_min); printf("%s ", pathname); } closedir(dir); return 0; }
这是一个简单的文件浏览器程序,可以列出指定目录下的所有文件和文件夹的详细信息,包括文件类型、权限、所有者、所属组、大小、创建时间和文件名等。程序的主要流程是先通过命令行参数获取要浏览的目录名,然后使用 opendir 函数打开该目录,使用 readdir 函数读取目录中的所有文件和文件夹,对于每个文件和文件夹,使用 lstat 函数获取其详细信息,然后打印出来。程序中还使用了一些系统函数和库函数,如 localtime、sprintf、strcmp 等。
阅读全文
相关推荐
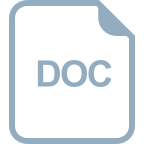
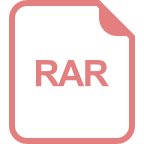
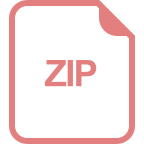





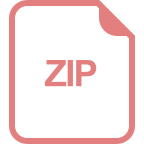
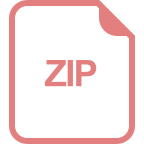
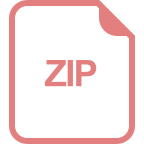




