#include <stdio.h> struct stu { int num; char sex; unsigned long birth; } stu_[3] = {{1, '1', 427}, {2, '2', 414}, {3, '2', 1212}}; struct stu *p = stu_; int main() { FILE *fp; fp = fopen("C:\Users\张px\Desktop\input.txt", "w+"); if (fp == NULL) { printf("Failed to open file.\n"); return 1; } if (fseek(fp, 0, SEEK_END) != 0) { printf("Failed to seek file.\n"); fclose(fp); return 1; } if (fwrite(p++, sizeof(struct stu), 3, fp) != 3) { printf("Failed to write data.\n"); fclose(fp); return 1; } fclose(fp); return 0; }写入文件的字符是乱码
时间: 2024-01-06 15:05:54 浏览: 25
你的代码中有几个问题:
1. 文件路径中的反斜杠需要转义,应该写成 "C:\\Users\\张px\\Desktop\\input.txt"
2. 在写入文件之前,应该将文件指针重新定位到文件开头,可以使用 fseek(fp, 0, SEEK_SET) 实现
3. 你的写入操作只会将结构体数组中的第一个元素写入文件,因为每次 p++ 后指向的是下一个结构体。你可以使用循环来写入所有的结构体。
修改后的代码如下所示:
```c
#include <stdio.h>
struct stu {
int num;
char sex;
unsigned long birth;
} stu_[3] = {{1, '1', 427}, {2, '2', 414}, {3, '2', 1212}};
struct stu *p = stu_;
int main() {
FILE *fp;
fp = fopen("C:\\Users\\张px\\Desktop\\input.txt", "wb+");
if (fp == NULL) {
printf("Failed to open file.\n");
return 1;
}
if (fseek(fp, 0, SEEK_SET) != 0) {
printf("Failed to seek file.\n");
fclose(fp);
return 1;
}
if (fwrite(stu_, sizeof(struct stu), 3, fp) != 3) {
printf("Failed to write data.\n");
fclose(fp);
return 1;
}
fclose(fp);
return 0;
}
```
注意,这里使用了二进制模式写入文件,因为结构体中包含二进制数据,如果使用文本模式会导致数据被转换成文本格式而出现乱码。
相关推荐
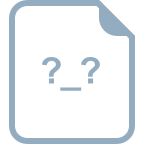
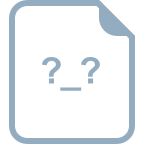
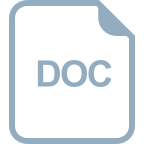








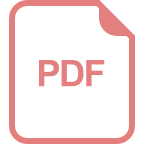
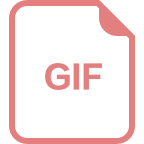
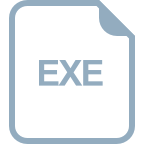
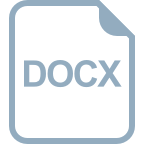