stb_image库的下载网址
时间: 2023-11-29 12:05:29 浏览: 52
stb_image 库的官方网址为 https://github.com/nothings/stb ,你可以在该网址下载最新版本的 stb_image.h 头文件。此外,stb_image.h 也被一些第三方网站提供下载,比如 https://github.com/foobar167/stb 、 https://github.com/nothings/stb/raw/master/stb_image.h 等等。请注意,下载的文件应该是 stb_image.h,而不是其他格式的文件。
相关问题
如何在自己的cmake里面添加stb_image库
在自己的 CMake 项目中添加 stb_image 库,可以按照以下步骤进行:
1. 将 stb_image.h 头文件复制到项目的 include 目录下(如果没有该目录,可以手动创建)。
2. 在 CMakeLists.txt 中,添加以下语句:
```
# 设置 stb_image 库的头文件路径
include_directories(${PROJECT_SOURCE_DIR}/include)
# 添加 stb_image 库的源文件
add_library(stb_image STATIC ${PROJECT_SOURCE_DIR}/src/stb_image.cpp)
```
其中,`${PROJECT_SOURCE_DIR}` 是指 CMake 项目的根目录,可以根据实际情况进行修改。`include_directories()` 指令用于设置头文件搜索路径,`${PROJECT_SOURCE_DIR}/include` 是 stb_image.h 头文件所在的路径;`add_library()` 指令用于添加库文件,`STATIC` 表示静态链接库,`${PROJECT_SOURCE_DIR}/src/stb_image.cpp` 是 stb_image 库的源文件路径,需要根据实际情况进行修改。
3. 在需要使用 stb_image 库的 C++ 文件中,添加以下语句:
```cpp
#include "stb_image.h"
```
这样就可以在项目中使用 stb_image 库了。注意,在编译项目时,需要链接 stb_image 库,可以在 CMakeLists.txt 中添加如下语句:
```
target_link_libraries(${PROJECT_NAME} stb_image)
```
其中,`${PROJECT_NAME}` 是指当前项目的名称,需要根据实际情况进行修改。
stb_image.h读取图片
stb_image.h 是一个非常简单易用的开源图像解码库,它可以读取多种格式的图片文件,包括 PNG、JPEG、BMP、GIF、PSD 等等。
使用 stb_image.h 读取图片非常简单,只需要包含头文件即可:
```c++
#define STB_IMAGE_IMPLEMENTATION
#include "stb_image.h"
```
然后调用 stbi_load 函数即可读取图片文件:
```c++
int width, height, channels;
unsigned char* image = stbi_load("test.png", &width, &height, &channels, STBI_RGB);
if (image != nullptr) {
// 使用 image 数据
stbi_image_free(image);
}
```
其中,第一个参数是图片文件的路径,第二个和第三个参数是指针,用来返回图片的宽度和高度,第四个参数是指针,用来返回图片的通道数。最后一个参数表示期望输出的颜色通道格式,可以是 STBI_grey、STBI_grey_alpha、STBI_RGB 或 STBI_RGBA。函数返回的是一个指向图片数据的 unsigned char 指针,如果读取失败则返回 nullptr。
需要注意的是,stbi_load 函数读取的数据是一个字节数组,每个像素的数据大小由通道数决定。例如,如果是 RGB 格式,则每个像素占用 3 个字节。因此,在使用 image 数据时,需要将其转换为正确的像素格式。
相关推荐
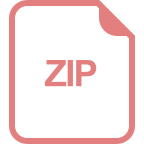











