c#datagridview将复选框选中的数据,将id列生成条码,型号列,日期列以文本的方式显示,通过brother打印机打印出来
时间: 2024-09-15 07:13:33 浏览: 63
要在 C# DataGridView 中实现这个功能,你可以按照以下步骤操作:
1. **选择复选框数据**:
通过事件监听 `CellContentClick`,找出用户点击的行,判断该行中的复选框是否选中。例如:
```csharp
private void dgv_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == dataGridView1.Columns["CheckboxColumn"].Index && e.RowIndex >= 0)
{
DataGridViewRow row = dgv.Rows[e.RowIndex];
bool isChecked = Convert.ToBoolean(row.Cells["CheckboxColumn"].Value);
if (isChecked)
{
// 获取选中的 ID、型号和日期
int id = Convert.ToInt32(row.Cells["IdColumn"].Value);
string model = row.Cells["ModelColumn"].Value.ToString();
DateTime date = Convert.ToDateTime(row.Cells["DateColumn"].Value);
// 处理生成条码等后续操作
GenerateBarcode(id);
}
}
}
```
2. **生成条码**:
使用第三方库如 `NHamcrest` 或 `Zxing.Net` 来生成条形码,这里仅示例使用字符串:
```csharp
private void GenerateBarcode(int id)
{
// 根据 id 生成条码字符串
string barcode = BarcodeGenerator.GenerateBarcode(id, BarcodeTypes.EAN_13); // 替换为你实际的生成函数
// 打印条码和信息
PrintToBrotherPrinter(barcode, model, date);
}
private void PrintToBrotherPrinter(string barcode, string model, DateTime date)
{
// 创建 Brother Printer 对象(假设你已经有了实例)
BrotherPrinter printer = GetBrotherPrinter();
// 构造要打印的信息
string text = $"ID: {id}\nModel: {model}\nDate: {date.ToString("yyyy-MM-dd")}\nBarcode: {barcode}";
// 打印内容到 Brother 打印机
printer.Print(text);
}
```
3. **连接 Brother 打印机**:
这部分依赖于具体的 Brother 打印机驱动和API,通常涉及到发送打印指令给打印机。你需要确保已安装正确的驱动并根据官方文档建立连接。
```csharp
private BrotherPrinter GetBrotherPrinter()
{
// 实现此处以获取 Brother 打印机对象
return new BrotherPrinter();
}
```
请注意,这只是一个基本的示例,实际实现可能需要调整以适应你的项目需求。同时,确保在打印过程中处理可能出现的异常,例如网络连接问题、打印机错误等。
阅读全文
相关推荐
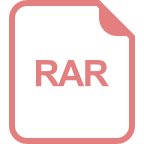
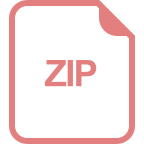
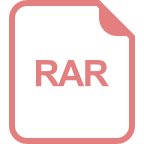
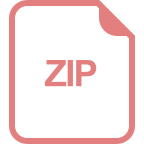
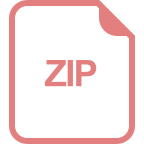
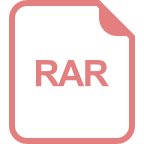





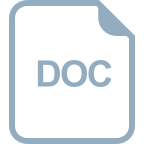
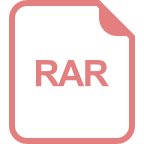
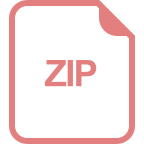
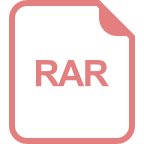
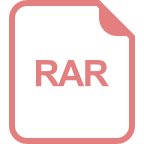
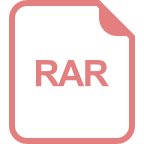
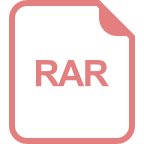
