PageInfo如何使用
时间: 2023-07-20 21:34:20 浏览: 96
使用PageInfo进行分页查询一般需要以下步骤:
1. 在Mapper接口中定义分页查询方法,方法参数为PageInfo类型对象和查询条件对象(例如实体类对象);
```java
public interface UserMapper {
List<User> selectUsers(PageInfo pageInfo, UserCondition condition);
}
```
2. 在Mapper映射文件中编写SQL语句,使用LIMIT关键字实现分页查询,并使用COUNT(*)语句查询总记录数;
```xml
<select id="selectUsers" resultType="com.example.User">
SELECT * FROM user
<where>
<if test="condition.name != null">
AND name = #{condition.name}
</if>
<!-- 其他查询条件 -->
</where>
ORDER BY create_time DESC
LIMIT #{pageInfo.offset}, #{pageInfo.pageSize}
</select>
<select id="selectUserCount" resultType="java.lang.Integer">
SELECT COUNT(*) FROM user
<where>
<if test="condition.name != null">
AND name = #{condition.name}
</if>
<!-- 其他查询条件 -->
</where>
</select>
```
3. 在Service层调用Mapper接口中的方法,传入PageInfo对象和查询条件对象,获取分页结果集;
```java
public class UserService {
@Autowired
private UserMapper userMapper;
public PageInfo<User> getUsers(int pageNum, int pageSize, UserCondition condition) {
PageInfo<User> pageInfo = new PageInfo<>(pageNum, pageSize);
List<User> userList = userMapper.selectUsers(pageInfo, condition);
pageInfo.setList(userList);
int total = userMapper.selectUserCount(condition);
pageInfo.setTotal(total);
return pageInfo;
}
}
```
4. 在Controller中接收请求参数,调用Service层方法进行分页查询,并将分页结果集传递给View层进行展示。
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/list")
public PageInfo<User> getUsers(@RequestParam(required = false, defaultValue = "1") int pageNum,
@RequestParam(required = false, defaultValue = "10") int pageSize,
UserCondition condition) {
return userService.getUsers(pageNum, pageSize, condition);
}
}
```
以上就是使用PageInfo进行分页查询的基本步骤,具体实现可以根据实际情况进行调整和优化。
阅读全文
相关推荐
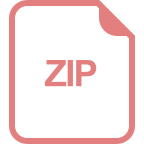
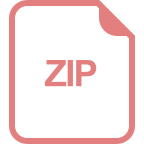
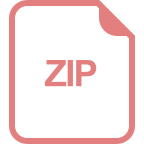















