创建 QTimer 定时器,每过一秒打印hi
时间: 2024-01-21 19:17:09 浏览: 40
好的,可以参考下面的代码实现:
```python
import sys
from PyQt5.QtWidgets import QApplication
from PyQt5.QtCore import QTimer
def print_hi():
print("hi")
if __name__ == '__main__':
app = QApplication(sys.argv)
# 创建 QTimer 定时器
timer = QTimer()
timer.timeout.connect(print_hi)
timer.start(1000) # 设置定时器间隔为 1 秒
sys.exit(app.exec_())
```
运行后,每隔一秒钟就会在控制台输出一次 “hi”。
相关问题
qt中定时器每隔一秒发送一次报文
在 Qt 中,可以使用 QTimer 类来创建定时器。为了每隔一秒发送一次报文,可以按照以下步骤操作:
1. 创建 QTimer 对象,并设置定时器的间隔为 1000ms(即1秒):
```cpp
QTimer *timer = new QTimer(this);
timer->setInterval(1000); // 设置定时器的间隔为1秒
```
2. 连接定时器的 timeout() 信号到槽函数,以便在定时器超时时发送报文:
```cpp
connect(timer, SIGNAL(timeout()), this, SLOT(sendMessage()));
```
其中,sendMessage() 是发送报文的槽函数。
3. 启动定时器:
```cpp
timer->start();
```
这样,定时器就会在启动后,每隔一秒触发一次 timeout() 信号,从而调用 sendMessage() 槽函数发送报文。
QTimer定时器使用
QTimer定时器是一个常用的计时器类,可以用于定时执行某些操作。使用QTimer定时器需要先创建一个QTimer对象,然后设置定时器的时间间隔和执行的槽函数,最后启动定时器即可。例如:
```c++
QTimer *timer = new QTimer(this); // 创建定时器对象
connect(timer, SIGNAL(timeout()), this, SLOT(mySlot())); // 设置定时器的槽函数
timer->start(1000); // 启动定时器,每隔1秒执行一次mySlot()函数
```
在槽函数中可以编写需要定时执行的代码,例如更新界面、发送网络请求等。需要注意的是,定时器的时间间隔不能太短,否则会影响程序的性能。
相关推荐
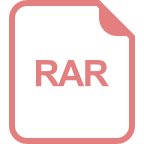












