vue点击更换背景图片
时间: 2023-10-10 22:10:36 浏览: 49
你可以使用v-bind绑定一个变量来控制背景图片的路径,在点击事件中更改这个变量的值。
例如:
HTML:
```
<div id="app" v-bind:style="{backgroundImage: 'url(' + backgroundImage + ')'}">
<button @click="changeBackground">Change Background</button>
</div>
```
JS:
```
new Vue({
el: '#app',
data: {
backgroundImage: 'https://example.com/background1.jpg'
},
methods: {
changeBackground: function() {
this.backgroundImage = 'https://example.com/background2.jpg';
}
}
})
```
这个例子中,初始背景图片是`background1.jpg`,当点击按钮时,会调用`changeBackground`方法,将背景图片更改为`background2.jpg`。
相关问题
vue盒子点击背景图片切换
你可以使用Vue的绑定语法 `:style` 和 `@click`,来实现点击盒子切换背景图片的效果。具体方法如下:
1. 在Vue组件的data中定义一个变量`bgIndex`,用于记录当前显示的背景图片的索引。并定义一组背景图片的数组`bgList`,用于存储不同的背景图片路径。
```
data() {
return {
bgIndex: 0,
bgList: [
'images/bg1.jpg',
'images/bg2.jpg',
'images/bg3.jpg'
]
}
}
```
2. 在Vue组件的模板中,使用`div`元素作为盒子,并绑定`style`属性,将背景图片路径设置为当前`bgIndex`所指向的图片。同时,给`div`元素绑定`@click`事件,当点击盒子时,执行`changeBg()`方法,切换背景图片。
```
<template>
<div class="box" :style="{ backgroundImage: 'url(' + bgList[bgIndex] + ')' }" @click="changeBg"></div>
</template>
```
3. 在Vue组件的方法中,定义`changeBg()`方法,用于切换背景图片。在该方法中,将`bgIndex`加1,如果`bgIndex`的值大于等于背景图片数组的长度,就将`bgIndex`重置为0,从而实现循环切换背景图片的效果。
```
methods: {
changeBg() {
this.bgIndex++;
if (this.bgIndex >= this.bgList.length) {
this.bgIndex = 0;
}
}
}
```
完整的Vue组件代码如下所示:
```
<template>
<div class="box" :style="{ backgroundImage: 'url(' + bgList[bgIndex] + ')' }" @click="changeBg"></div>
</template>
<script>
export default {
data() {
return {
bgIndex: 0,
bgList: [
'images/bg1.jpg',
'images/bg2.jpg',
'images/bg3.jpg'
]
}
},
methods: {
changeBg() {
this.bgIndex++;
if (this.bgIndex >= this.bgList.length) {
this.bgIndex = 0;
}
}
}
}
</script>
<style scoped>
.box {
width: 500px;
height: 300px;
cursor: pointer;
transition: background-image 0.5s ease-in-out;
}
</style>
```
vue点击按钮更换背景图片
您可以通过以下步骤实现Vue中点击按钮更换背景图片:
1. 将需要更换的背景图片存储在Vue组件的data中,例如:
```javascript
data() {
return {
backgroundImage: 'url(https://example.com/background1.jpg)'
}
}
```
2. 在Vue组件中添加一个按钮,并为其绑定一个点击事件,例如:
```html
<template>
<div>
<button @click="changeBackground">更换背景图片</button>
<div :style="{ backgroundImage }"></div>
</div>
</template>
```
3. 在Vue组件的methods中添加changeBackground方法,该方法将在点击按钮时触发,并更新组件的data中的背景图片:
```javascript
methods: {
changeBackground() {
this.backgroundImage = 'url(https://example.com/background2.jpg)'
}
}
```
4. 最后,您可以通过CSS样式来设置背景图片的尺寸和其他样式,例如:
```css
div {
background-size: cover;
background-position: center;
width: 100%;
height: 100vh;
}
```
相关推荐
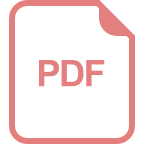
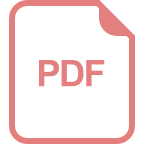












