vue quill 撤销
时间: 2023-09-16 22:06:34 浏览: 171
### 回答1:
Vue Quill组件默认提供了撤销和重做的功能,你可以通过在Quill实例上调用`undo()`和`redo()`方法来实现撤销和重做操作。同时,你也可以通过在Quill组件上添加`@keydown`事件监听器来捕获用户的撤销和重做快捷键(如Ctrl+Z和Ctrl+Shift+Z)的按下事件,并在这些事件发生时手动调用`undo()`和`redo()`方法。以下是一个示例代码:
```html
<template>
<quill-editor v-model="content" @keydown.native.ctrl.z.prevent="undo" @keydown.native.ctrl.shift.z.prevent="redo" />
</template>
<script>
import { quillEditor } from 'vue-quill-editor'
export default {
components: {
quillEditor
},
data () {
return {
content: ''
}
},
methods: {
undo () {
this.$refs.quillEditor.quill.history.undo()
},
redo () {
this.$refs.quillEditor.quill.history.redo()
}
}
}
</script>
```
在这个示例中,我们使用了Vue Quill组件的`v-model`指令来双向绑定编辑器的内容。我们还在Quill组件上添加了`@keydown`事件监听器,并分别捕获了Ctrl+Z和Ctrl+Shift+Z的按下事件。在`undo()`和`redo()`方法中,我们通过`this.$refs.quillEditor.quill.history.undo()`和`this.$refs.quillEditor.quill.history.redo()`来手动调用Quill实例的撤销和重做方法。
### 回答2:
Vue Quill是一个基于Vue.js的富文本编辑器组件,它提供了丰富的功能和风格自定义的选项。在Vue Quill中,撤销操作的实现相对简单。
在Vue Quill中,撤销操作可以通过Quill实例的undo()方法来实现。当用户执行撤销操作时,可以在需要的地方监听相应的事件,然后调用Quill实例的undo()方法即可实现撤销功能。
以下是一个简单的示例代码,展示如何在Vue Quill中实现撤销操作:
```
<template>
<div>
<div ref="editor" v-model="content"></div>
<button @click="undo">撤销</button>
</div>
</template>
<script>
import { quillEditor } from 'vue-quill-editor'
export default {
components: {
quillEditor
},
data() {
return {
content: '',
quillInstance: null
}
},
mounted() {
this.$refs.editor.quill.on('text-change', () => {
this.content = this.quillInstance.root.innerHTML
})
this.quillInstance = this.$refs.editor.quill
},
methods: {
undo() {
this.quillInstance.history.undo()
}
}
}
</script>
```
在上述代码中,我们首先在`<div ref="editor" v-model="content"></div>`中渲染Vue Quill编辑器,并将其值绑定到`content`属性上。
然后,在`mounted`生命周期钩子中,我们监听了Vue Quill的`text-change`事件,并将编辑器内容的变化同步到`content`属性中,以便实现撤销操作。
最后,在按钮的`click`事件中调用`undo`方法,该方法利用Quill实例的`history`对象的`undo()`方法实现撤销操作。
在这个简单的示例中,我们可以通过点击“撤销”按钮来撤销我们在编辑器中的操作,实现了撤销功能。
### 回答3:
Vue Quill是一个基于Quill富文本编辑器的Vue组件,它提供了一些额外的功能和样式,使得在Vue项目中使用Quill更加方便。
要实现撤销功能,我们可以借助Quill编辑器本身提供的API。Quill编辑器有一个`history`插件,它内置了一些用于管理撤销和恢复操作的方法。
首先,我们需要在Vue Quill的配置中加入`history`插件,可以使用`toolbar`选项来添加一个撤销按钮:
```
Vue.use(VueQuillEditor, {
modules: {
toolbar: [
['bold', 'italic', 'underline', 'strike'],
['blockquote', 'code-block'],
[{ 'header': 1 }, { 'header': 2 }],
[{ 'list': 'ordered' }, { 'list': 'bullet' }],
[{ 'script': 'sub' }, { 'script': 'super' }],
[{ 'indent': '-1' }, { 'indent': '+1' }],
[{ 'direction': 'rtl' }],
[{ 'size': ['small', false, 'large', 'huge'] }],
[{ 'header': [1, 2, 3, 4, 5, 6, false] }],
[{ 'color': [] }, { 'background': [] }],
[{ 'font': [] }],
[{ 'align': [] }],
['link', 'image', 'video', 'formula'],
['clean'],
['undo', 'redo'] // 添加撤销和重做按钮
],
history: {
delay: 2000, // 撤销的时间间隔
maxStack: 500, // 最多保存的操作步骤数
userOnly: false // 是否只保存用户的操作步骤
}
},
// 其他配置...
})
```
配置完毕后,我们就可以在Quill编辑器中看到撤销按钮了。
在代码中使用撤销功能很简单,我们可以通过编辑器实例的`history`属性来调用相应的方法。例如,要撤销最近一次的操作,可以使用`editor.history.undo()`方法;要恢复被撤销的操作,可以使用`editor.history.redo()`方法。
```javascript
// Vue组件中的代码
<template>
<div>
<vue-quill-editor v-model="content"></vue-quill-editor>
<button @click="undo">撤销</button>
<button @click="redo">恢复</button>
</div>
</template>
<script>
export default {
data() {
return {
content: '',
editor: null // 保存编辑器实例
}
},
mounted() {
// 实例化编辑器后保存实例
this.editor = this.$refs.myQuillEditor.quill
},
methods: {
undo() {
this.editor.history.undo()
},
redo() {
this.editor.history.redo()
}
}
}
</script>
```
以上就是利用Vue Quill的配置和Quill编辑器的`history`插件来实现撤销功能的简单示例。通过添加撤销和重做按钮,并结合编辑器实例的`history`属性,我们可以方便地管理用户的操作步骤,使得撤销和恢复操作变得简单易用。
阅读全文
相关推荐
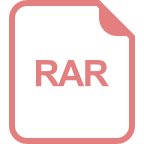
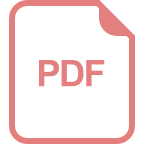
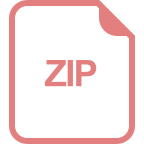








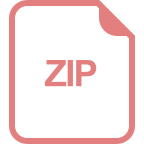
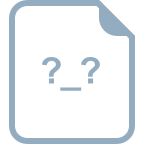
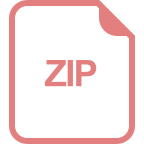
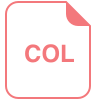


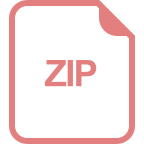