import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int m = sc.nextInt(); int n = sc.nextInt(); int[][] matrix = new int[m][n]; for (int i = 0; i < m; i++) { for (int j = 0; j < n; j++) { matrix[i][j] = sc.nextInt(); } } System.out.println(getResult(m, n, matrix)); } public static int getResult(int m, int n, int[][] matrix) { int count = 0; int[][] offsets = {{-1, 0}, {1, 0}, {0, 1}, {0, -1}}; for (int x = 0; x < m; x++) { for (int y = 0; y < n; y++) { if (matrix[x][y] == 1) { count++; continue; } for (int[] offset : offsets) { int newX = x + offset[0]; int newY = y + offset[1]; if (newX >= 0 && newX < m && newY >= 0 && newY < n && matrix[newX][newY] == 1) { count++; break; } } } } return count; } }翻译成C++
时间: 2024-04-14 16:27:49 浏览: 126
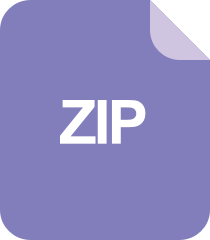
java代码-实训4-3 import java.util.Scanner
以下是将Java代码翻译成C++的版本:
```cpp
#include <iostream>
#include <vector>
using namespace std;
int getResult(int m, int n, vector<vector<int>>& matrix) {
int count = 0;
vector<vector<int>> offsets = {{-1, 0}, {1, 0}, {0, 1}, {0, -1}};
for (int x = 0; x < m; x++) {
for (int y = 0; y < n; y++) {
if (matrix[x][y] == 1) {
count++;
continue;
}
for (auto offset : offsets) {
int newX = x + offset[0];
int newY = y + offset[1];
if (newX >= 0 && newX < m && newY >= 0 && newY < n && matrix[newX][newY] == 1) {
count++;
break;
}
}
}
}
return count;
}
int main() {
int m, n;
cin >> m >> n;
vector<vector<int>> matrix(m, vector<int>(n));
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
cin >> matrix[i][j];
}
}
cout << getResult(m, n, matrix) << endl;
return 0;
}
```
这个C++版本的代码与你提供的Java代码功能相同,使用了 `vector` 容器来表示二维数组,并使用嵌套的 `for` 循环来遍历和计算结果。在 `getResult` 函数中,我们遵循了相同的逻辑来统计与值为1的元素相邻的元素个数。
阅读全文
相关推荐
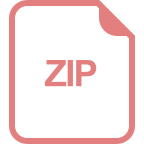













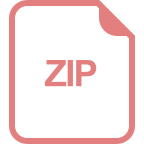
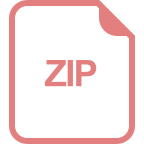
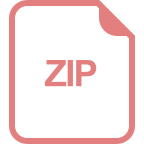