在C语言中如何利用链表结构实现学生信息管理系统,并通过磁盘文件实现数据持久化?
时间: 2024-11-14 17:38:11 浏览: 4
要实现一个学生信息管理系统,首先需要掌握链表的基本操作,包括创建节点、插入节点、删除节点以及遍历链表。这里我们可以定义一个学生信息的结构体,包括学号、姓名和籍贯等字段,并将每个学生信息封装成一个链表节点。以下是详细的实现步骤:
参考资源链接:[C语言课程设计:学生籍贯信息管理系统](https://wenku.csdn.net/doc/4uwdox00kx?spm=1055.2569.3001.10343)
1. **定义学生信息结构体和链表节点**:
```c
typedef struct {
int id; // 学号
char name[50]; // 姓名
char origin[50]; // 籍贯
} StudentInfo;
typedef struct Node {
StudentInfo data; // 存储学生信息
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. **创建链表**:
创建链表涉及到创建头节点,并能够根据用户输入添加新的节点到链表中。
```c
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node));
if (head != NULL) {
head->next = NULL;
}
return head;
}
```
3. **添加学生信息到链表**:
通过输入学生信息并创建新节点,然后将新节点插入到链表的适当位置。
```c
void addStudentInfo(Node* head, StudentInfo info) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = info;
newNode->next = head->next;
head->next = newNode;
}
}
```
4. **删除链表中的学生信息**:
通过学号来找到对应的节点并删除。
```c
void deleteStudentInfo(Node* head, int id) {
Node* current = head;
while (current->next != NULL && current->next->data.id != id) {
current = current->next;
}
if (current->next != NULL) {
Node* temp = current->next;
current->next = temp->next;
free(temp);
}
}
```
5. **修改链表中的学生信息**:
类似删除操作,找到节点后修改信息。
```c
void updateStudentInfo(Node* head, int id, StudentInfo newInfo) {
Node* current = head->next;
while (current != NULL && current->data.id != id) {
current = current->next;
}
if (current != NULL) {
current->data = newInfo;
}
}
```
6. **查询链表中的学生信息**:
根据学号或姓名查询学生信息,并通过打印的方式显示。
```c
void searchStudentInfo(Node* head, int id) {
Node* current = head->next;
while (current != NULL) {
if (current->data.id == id) {
printStudentInfo(current->data);
return;
}
current = current->next;
}
printf(
参考资源链接:[C语言课程设计:学生籍贯信息管理系统](https://wenku.csdn.net/doc/4uwdox00kx?spm=1055.2569.3001.10343)
阅读全文
相关推荐
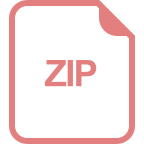
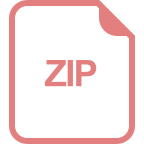
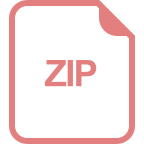

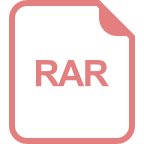
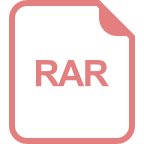
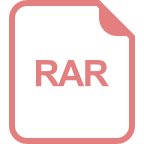
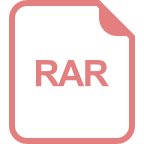
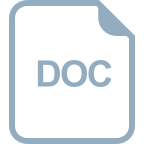
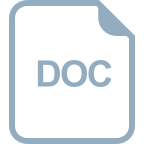
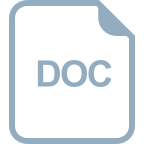
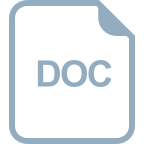
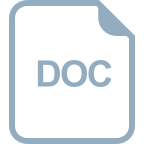
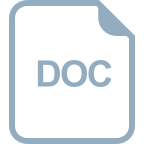
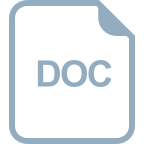
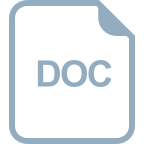

