为学生数据库管理系统编写 C++ 程序,完整可运行,中文,没有报错
时间: 2024-10-11 07:14:14 浏览: 18
创建一个完整的可运行的学生数据库管理系统(SDBMS)是一个复杂的任务,需要涉及数据结构、文件操作、命令行界面以及数据库查询等功能。下面是一个简化的 C++ 示例,展示如何设计一个基本的学生信息存储和检索功能。请注意,这个例子只是一个基础框架,实际的 SDBMS 应该包含更多的功能和错误处理。
```cpp
#include <iostream>
#include <fstream>
#include <string>
struct Student {
std::string name;
int id;
float grade;
};
class Database {
private:
std::ofstream db_file; // 学生数据库文件
public:
void open(const std::string& filename) {
if (db_file.is_open()) {
db_file.close();
}
db_file.open(filename, std::ios::app);
if (!db_file.is_open()) {
throw std::runtime_error("无法打开数据库文件");
}
}
void addStudent(Student student) {
db_file << "Name: " << student.name << ", ID: " << student.id << ", Grade: " << student.grade << "\n";
}
void searchStudent(int id) {
db_file.seekg(0, std::ios::beg); // 从头开始读取
while (true) {
std::string line;
getline(db_file, line);
if (line.empty()) break;
// 解析每一行
auto fields = line.split(',');
if (std::stoi(fields[1]) == id) {
std::cout << "Found student with ID: " << id << "\n";
std::cout << "Name: " << fields[0] << ", Grade: " << fields[2] << "\n";
return;
}
}
std::cout << "No student found with ID: " << id << "\n";
}
};
int main() {
Database db("students.txt");
try {
db.open("students.txt");
db.addStudent({ "张三", 1001, 90.5 });
db.addStudent({ "李四", 1002, 85.0 });
// 搜索特定学生
db.searchStudent(1001);
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
阅读全文
相关推荐
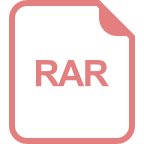
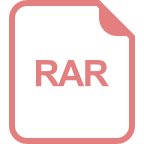
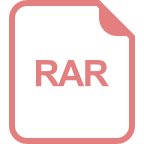















