c++,用指针字符串复制代码
时间: 2024-09-26 12:05:24 浏览: 51
在C++中,复制字符串通常使用指针操作,特别是当涉及到字符数组时。经典的C++方式是通过指针来逐个元素复制,这里有一个简单的示例:
```cpp
#include <cstring> // 包含strcpy函数
// 定义两个字符数组(字符串)
char source[] = "Hello, World!";
char destination[20]; // 假设足够大能容纳源字符串
// 使用strcpy函数复制字符串
strcpy(destination, source);
// 注意:strncpy也可以用于防止溢出,但需要指定目的字符串的最大长度
// char destination[16];
// strncpy(destination, source, sizeof(destination));
// 检查复制是否成功
if (destination[sizeof(source) - 1] == '\0') {
std::cout << "String copied successfully!" << std::endl;
} else {
std::cerr << "String copy failed!" << std::endl;
}
相关问题
c++用指针实现字符串的复制
C++中可以使用指针来实现字符串的复制。下面是一个示例代码:
```cpp
#include <iostream>
using namespace std;
void stringCopy(char* dest, const char* src) {
while (*src != '\0') {
*dest = *src;
dest++;
src++;
}
*dest = '\0';
}
int main() {
char str1[] = "I love CHINA!";
char str2[20];
stringCopy(str2, str1);
cout << "str1 is: " << str1 << endl;
cout << "str2 is: " << str2 << endl;
return 0;
}
```
这段代码中,我们定义了一个`stringCopy`函数,它接受两个参数:目标字符串的指针`dest`和源字符串的指针`src`。函数通过循环将源字符串中的每个字符复制到目标字符串中,直到遇到字符串结束符`\0`。最后,我们在`main`函数中调用`stringCopy`函数来复制字符串,并输出结果。
用c++的指针编程:使用字符型指针复制字符串。要代码和结果
下面是一个使用字符型指针复制字符串的 C++ 代码示例:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
char str1[] = "Hello, world!"; // 原始字符串
char *str2; // 指向新字符串的指针
// 复制字符串
str2 = new char[strlen(str1) + 1];
strcpy(str2, str1);
// 输出结果
cout << "原始字符串为:" << str1 << endl;
cout << "新字符串为:" << str2 << endl;
delete[] str2; // 释放内存
return 0;
}
```
代码输出结果如下:
```
原始字符串为:Hello, world!
新字符串为:Hello, world!
```
在这个示例中,我们首先定义了一个字符数组 `str1`,其中包含原始字符串。然后,我们声明了一个字符型指针 `str2`,该指针将用于指向新字符串。接下来,我们使用 `new` 运算符为 `str2` 动态分配了足够的内存,以容纳 `str1` 中的字符串。然后,我们使用 `strcpy` 函数将 `str1` 中的字符串复制到 `str2` 中。最后,我们输出了原始字符串和复制后的字符串,并使用 `delete[]` 运算符释放了 `str2` 所指向的内存。
需要注意的是,在动态分配内存时,我们必须记得释放这些内存以避免内存泄漏。在本示例中,我们使用了 `delete[]` 运算符来释放 `str2` 所指向的内存。
阅读全文
相关推荐







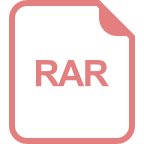








