编写一个学生和职工数据输入和输出程序,学生数据要有学号、姓名、性别、专业、年级等,职工数据要有职工号、姓名、性别、年龄、部门、职称、工资等。要求将姓名、性别和数据输出设计成一个类person,学生类student和职工类employee,由类person来派生。要求程序完整(包含主函数)
时间: 2023-06-15 07:04:58 浏览: 111
以下是一个简单的C++程序,实现了学生和职工数据的输入和输出:
```cpp
#include <iostream>
#include <string>
using namespace std;
class person {
protected:
string name;
char sex;
public:
virtual void input() {
cout << "姓名:";
cin >> name;
cout << "性别:";
cin >> sex;
}
virtual void output() {
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
}
};
class student : public person {
private:
string id;
string major;
int grade;
public:
void input() {
person::input();
cout << "学号:";
cin >> id;
cout << "专业:";
cin >> major;
cout << "年级:";
cin >> grade;
}
void output() {
person::output();
cout << "学号:" << id << endl;
cout << "专业:" << major << endl;
cout << "年级:" << grade << endl;
}
};
class employee : public person {
private:
string id;
int age;
string department;
string title;
double salary;
public:
void input() {
person::input();
cout << "职工号:";
cin >> id;
cout << "年龄:";
cin >> age;
cout << "部门:";
cin >> department;
cout << "职称:";
cin >> title;
cout << "工资:";
cin >> salary;
}
void output() {
person::output();
cout << "职工号:" << id << endl;
cout << "年龄:" << age << endl;
cout << "部门:" << department << endl;
cout << "职称:" << title << endl;
cout << "工资:" << salary << endl;
}
};
int main() {
student stu;
employee emp;
cout << "请输入学生信息:" << endl;
stu.input();
cout << "请输入职工信息:" << endl;
emp.input();
cout << "学生信息如下:" << endl;
stu.output();
cout << "职工信息如下:" << endl;
emp.output();
return 0;
}
```
在程序中定义了一个person类作为基类,包含了姓名和性别两个成员变量以及输入输出函数。然后分别定义了student类和employee类,均继承自person类,并增加了各自的成员变量和输入输出函数。在main函数中,先创建一个学生对象和一个职工对象,然后通过对象调用各自的输入输出函数即可。
相关推荐
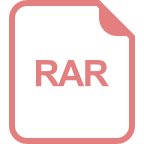
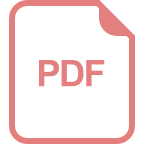
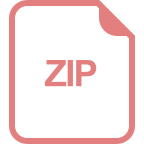
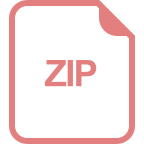












