vue3+ts的tab栏怎么做
时间: 2023-12-21 11:05:46 浏览: 29
Vue3+TypeScript的Tab栏可以使用Vue3的Composition API和Vue3自带的Tab组件来实现。
首先,需要定义Tab栏的数据结构,如下所示:
```typescript
interface Tab {
id: number;
title: string;
content: string;
}
```
然后,需要定义Tab栏组件的Props和数据:
```typescript
import { defineComponent, PropType, ref } from 'vue';
export default defineComponent({
props: {
tabs: {
type: Array as PropType<Tab[]>,
required: true,
},
},
setup(props) {
// 当前选中的Tab
const activeTab = ref(props.tabs[0].id);
// 切换Tab
const switchTab = (id: number) => {
activeTab.value = id;
};
return {
activeTab,
switchTab,
};
},
});
```
然后,在Tab栏组件中,使用Vue3自带的Tab组件来渲染Tab栏:
```html
<template>
<div class="tabs">
<div class="tab-nav">
<tab-nav v-model="activeTab">
<template v-for="tab in tabs">
<tab-pane :label="tab.title" :name="tab.id.toString()" :key="tab.id">
{{ tab.content }}
</tab-pane>
</template>
</tab-nav>
</div>
</div>
</template>
<script lang="ts">
import { defineComponent, PropType, ref } from 'vue';
interface Tab {
id: number;
title: string;
content: string;
}
export default defineComponent({
props: {
tabs: {
type: Array as PropType<Tab[]>,
required: true,
},
},
setup(props) {
// 当前选中的Tab
const activeTab = ref(props.tabs[0].id);
// 切换Tab
const switchTab = (id: number) => {
activeTab.value = id;
};
return {
activeTab,
switchTab,
};
},
});
</script>
```
最后,在父组件中使用Tab栏组件来渲染Tab栏:
```html
<template>
<div>
<my-tabs :tabs="tabs"></my-tabs>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
import MyTabs from './MyTabs.vue';
export default defineComponent({
components: {
MyTabs,
},
data() {
return {
tabs: [
{
id: 1,
title: 'Tab 1',
content: 'Content of Tab 1',
},
{
id: 2,
title: 'Tab 2',
content: 'Content of Tab 2',
},
{
id: 3,
title: 'Tab 3',
content: 'Content of Tab 3',
},
],
};
},
});
</script>
```
这样,就完成了Vue3+TypeScript的Tab栏的实现。
相关推荐
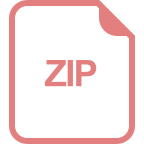
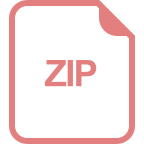














