python多线程 如何从mqtt服务器多个消息队列拿数据,请以代码示例
时间: 2024-03-15 19:46:46 浏览: 154
好的,以下是一个使用 Python 多线程从 MQTT 服务器多个消息队列中获取数据的示例代码:
```python
import threading
import paho.mqtt.client as mqtt
class MqttSubscriber(threading.Thread):
def __init__(self, broker_url, topic):
threading.Thread.__init__(self)
self.broker_url = broker_url
self.topic = topic
self.client = mqtt.Client()
def run(self):
def on_message(client, userdata, message):
"""MQTT消息回调函数"""
print(f"Received message on topic {message.topic}: {message.payload.decode()}")
self.client.on_message = on_message
self.client.connect(self.broker_url)
self.client.subscribe(self.topic)
self.client.loop_forever()
if __name__ == "__main__":
# 创建多个MqttSubscriber线程
threads = []
topics = ["topic1", "topic2", "topic3"]
for topic in topics:
t = MqttSubscriber("mqtt.broker.com", topic)
threads.append(t)
# 启动所有线程
for t in threads:
t.start()
# 等待所有线程执行完毕
for t in threads:
t.join()
print("All threads are finished")
```
这个示例程序创建了多个 `MqttSubscriber` 线程,每个线程订阅一个 MQTT 消息队列,并在回调函数中输出消息。在主线程中,启动了所有线程,然后等待所有线程执行完毕。最后输出所有线程都完成的信息。
总结一下 Python 多线程从 MQTT 服务器多个消息队列中获取数据的几个要点:
1. 使用 `paho.mqtt.client` 模块连接 MQTT 服务器,并订阅消息队列。
2. 在回调函数中处理接收到的消息。
3. 使用 `threading.Thread` 创建多个线程,每个线程订阅一个消息队列。
4. 使用 `start` 方法启动线程。
5. 使用 `join` 方法等待所有线程执行完毕。
阅读全文
相关推荐
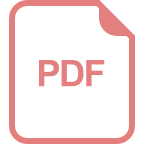
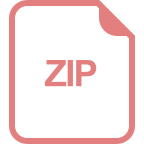
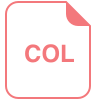
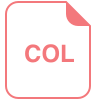
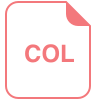
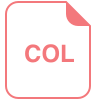
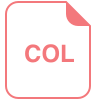
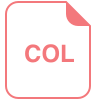
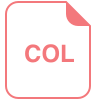
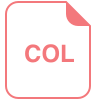
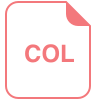
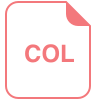
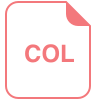
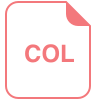
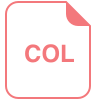
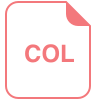
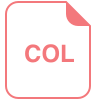