#include <iostream> #include <sstream> #include <string> #include <vector> #include <utility> #include <algorithm> using namespace std; class Solution { public: // 待实现函数,在此函数中填入答题代码; string GetTaggedString(const vector<string>& words, const string& inputStr) { string result; vector<vector<int>> sheet; for (int i = 0; i < inputStr.length();; i++) { for (int j = 0; j < i; j++) { auto currStr = inputStr.substr(j, i - j + 1); if (find(words.begin(), words.end(), currStr) != words.end()) { vector curr = { j, i }; sheet.push_back(curr); } } } return result; } }; inline int ReadInt() { int number; std::cin >> number; return number; } void SplitString(const string& input, char sperChar, vector<string>& outArray) { stringstream sstr(input); string token; while (getline(sstr, token, sperChar)) { outArray.push_back(token); } } inline string ReadLine() { string line; getline(cin, line); return line; } int main() { int wordsNum = ReadInt(); cin.ignore(); vector<string> words; string wordsList = ReadLine(); SplitString(wordsList, ' ', words); words.resize(wordsNum); string inputStr = ReadLine(); Solution solu; string result = solu.GetTaggedString(words, inputStr); cout << result; return 0; }
时间: 2024-02-15 07:28:39 浏览: 84
这段代码是一个 C++ 程序,用于读取用户输入的一些字符串和单词,并将这些单词在字符串中的位置标记出来。
其中,类 Solution 中的 GetTaggedString 函数是需要实现的部分。它接受两个参数,一个是单词列表 words,另一个是输入字符串 inputStr。函数的作用是将输入字符串 inputStr 中包含在单词列表 words 中的单词标记出来,并返回一个新的字符串 result,其中标记的方式是用一对尖括号 < > 将单词包围起来。
具体实现的方法是,遍历字符串 inputStr 中的所有子串,如果子串是单词列表中的一个单词,则将该子串的起始位置和结束位置记录下来,并保存在一个名为 sheet 的二维数组中。然后,对于每个单词列表中的单词,在 inputStr 中找到所有出现该单词的位置,并将这些位置转换成标记,最终生成新的字符串 result。
main 函数负责从标准输入读取数据,并调用 GetTaggedString 函数进行处理,并将结果输出到标准输出。
注意,这段代码仅仅是一个框架,需要根据题目的具体要求进行修改和完善才能得到正确的结果。
相关问题
#include<bits/stdc++.h>包含哪些头文件
这个头文件包含了所有标准的 C++ 库头文件,包括一些常用的输入输出、字符串处理、数学计算、文件操作等功能的头文件。具体包含的头文件如下:
- iostream:输入输出流
- sstream:字符串流
- cmath:数学函数
- cstdio:输入输出流
- cstdlib:标准库函数
- cctype:字符处理函数
- string:字符串相关
- algorithm:算法相关
- vector:向量相关
- queue:队列相关
- stack:栈相关
- deque:双端队列相关
- list:链表相关
- set:集合相关
- map:映射相关
- unordered_set:无序集合相关
- unordered_map:无序映射相关
- functional:函数对象相关
- iterator:迭代器相关
- utility:工具函数相关
阅读全文
相关推荐
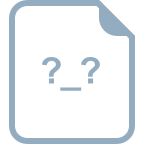
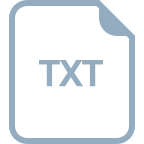
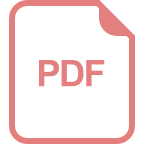
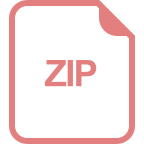
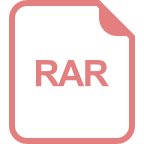
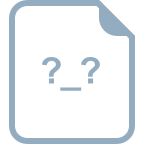
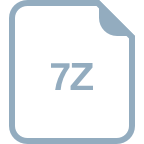
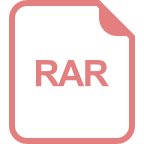