在 main 方法中从键盘输入两个整数,依次为:随机种子 seed 和 复数类 Complex 对象的个数 num 。然后随机生成 num 个 Complex 对象存入 cs1 数组中,再随机生成 num 个 Complex 对象存入 cs2 数组中。 然后对 cs1 和 cs2 数组中对应的复数对象依次执行如下操作: ① 判断 cs1[i] 和 cs2[i] 是否相等; ② 将 cs1[i] 和 cs2[i] 相加并输出; ③ 将 cs1[i] 和 cs2[i] 相减并输出; ④ 将 cs1[
时间: 2023-06-17 07:03:31 浏览: 137
i] 和 cs2[i] 相乘并输出。下面是代码实现:
```java
import java.util.Scanner;
import java.util.Random;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("请输入随机种子:");
int seed = input.nextInt();
System.out.print("请输入复数对象的个数:");
int num = input.nextInt();
Random random = new Random(seed);
Complex[] cs1 = new Complex[num];
Complex[] cs2 = new Complex[num];
// 随机生成复数对象并存入数组中
for (int i = 0; i < num; i++) {
double real = random.nextDouble() * 10;
double imag = random.nextDouble() * 10;
cs1[i] = new Complex(real, imag);
real = random.nextDouble() * 10;
imag = random.nextDouble() * 10;
cs2[i] = new Complex(real, imag);
}
// 依次执行操作
for (int i = 0; i < num; i++) {
System.out.println("第" + (i+1) + "对复数:");
if (cs1[i].equals(cs2[i])) {
System.out.println("cs1[" + i + "] 和 cs2[" + i + "] 相等");
} else {
System.out.println("cs1[" + i + "] 和 cs2[" + i + "] 不相等");
}
Complex addResult = cs1[i].add(cs2[i]);
System.out.println("cs1[" + i + "] + cs2[" + i + "] = " + addResult);
Complex subResult = cs1[i].sub(cs2[i]);
System.out.println("cs1[" + i + "] - cs2[" + i + "] = " + subResult);
Complex mulResult = cs1[i].mul(cs2[i]);
System.out.println("cs1[" + i + "] * cs2[" + i + "] = " + mulResult);
}
}
}
class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public boolean equals(Complex c) {
return this.real == c.real && this.imag == c.imag;
}
public Complex add(Complex c) {
return new Complex(this.real + c.real, this.imag + c.imag);
}
public Complex sub(Complex c) {
return new Complex(this.real - c.real, this.imag - c.imag);
}
public Complex mul(Complex c) {
double real = this.real * c.real - this.imag * c.imag;
double imag = this.real * c.imag + this.imag * c.real;
return new Complex(real, imag);
}
public String toString() {
return String.format("%.2f+%.2fi", real, imag);
}
}
```
阅读全文
相关推荐
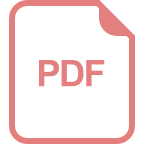
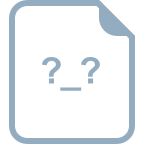
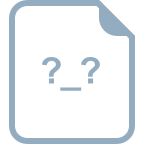
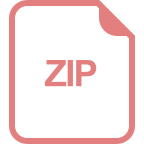
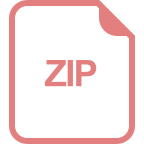
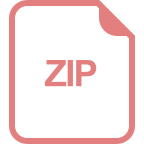
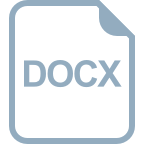
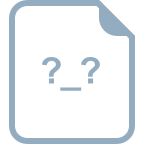
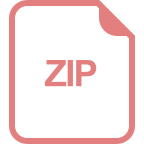
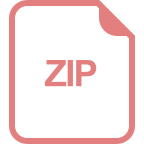
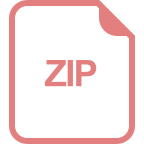
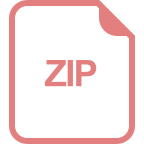
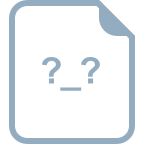
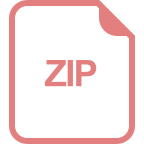
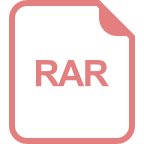
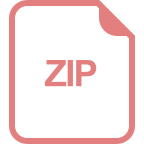
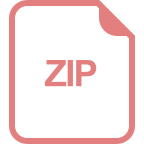
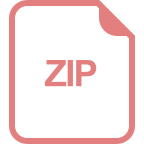
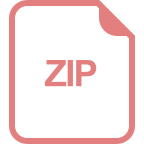