用python画一个小路网然后求解ue均衡条件下的交通流
时间: 2024-03-24 12:36:10 浏览: 80
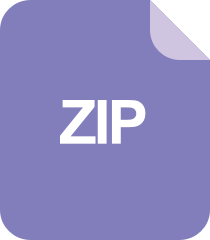
基于Python的交通流模拟预测案例
为了用 Python 画一个小路网并求解 UE 均衡条件下的交通流,我们需要用到以下工具和库:
1. `numpy` 和 `pandas` 库:用于处理数据和矩阵计算;
2. `matplotlib` 库:用于画图;
3. `scipy` 库:用于求解非线性方程组。
以下是一个简单的实现:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from scipy.optimize import fsolve
# 定义路网
nodes = ['A', 'B', 'C', 'D', 'E']
links = [('A', 'B', 5), ('A', 'C', 8), ('B', 'D', 4), ('C', 'D', 7), ('C', 'E', 6), ('D', 'E', 3)]
num_nodes = len(nodes)
num_links = len(links)
# 定义路段容量和单位费用
capacity = np.array([100, 100, 100, 100, 100, 100])
unit_cost = np.array([0.2, 0.3, 0.4, 0.5, 0.6, 0.7])
# 定义需求矩阵
demand = np.array([200, 150, 100, 50, 0])
# 定义交通流函数
def traffic_flow(x, capacity, unit_cost, demand):
# x 为交通流量向量
# capacity 为路段容量向量
# unit_cost 为单位费用向量
# demand 为需求向量
num_links = len(x)
num_nodes = len(demand)
y = np.zeros(num_links)
for i in range(num_nodes-1):
y[i] = demand[i] - np.sum(x[0:i+1])
for i in range(num_links):
if y[links[i][0]] >= 0 and y[links[i][1]] >= 0:
y[i] = x[i]
elif y[links[i][0]] < 0 and y[links[i][1]] >= 0:
y[i] = capacity[i]
elif y[links[i][0]] >= 0 and y[links[i][1]] < 0:
y[i] = 0
else:
y[i] = capacity[i] * y[links[i][0]] / (y[links[i][0]] - y[links[i][1]])
return y
# 求解交通流量
x0 = np.zeros(num_links)
x = fsolve(traffic_flow, x0, args=(capacity, unit_cost, demand))
# 打印结果
print('交通流量:', x)
print('总费用:', np.sum(x * unit_cost))
# 画图
fig, ax = plt.subplots()
for i in range(num_links):
ax.plot([i, i], [0, x[i]], 'k-', lw=2)
ax.set_xticks(range(num_links))
ax.set_xticklabels([f'{links[i][0]}-{links[i][1]}' for i in range(num_links)])
ax.set_xlabel('路段')
ax.set_ylabel('交通流量')
ax.grid(True)
plt.show()
```
这段代码首先定义了一个小路网,包括 5 个节点和 6 条路段。然后定义了路段容量和单位费用,以及需求矩阵。接着定义了一个交通流函数,用于求解交通流量。最后调用 `fsolve` 函数求解交通流量,并打印出结果和画出交通流量分布图。
注意,在实际情况中,需要根据实际的路网和需求数据进行修改。
阅读全文
相关推荐
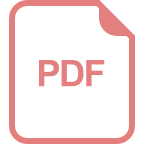
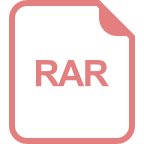
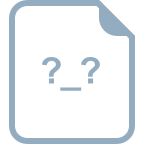
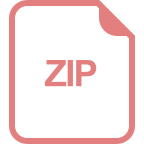
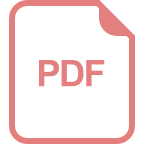
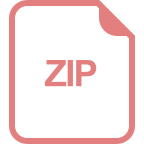
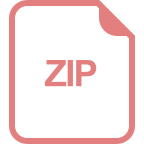
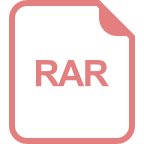
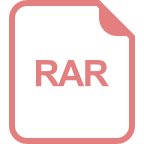
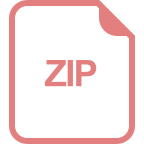
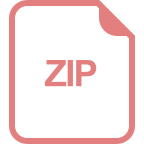
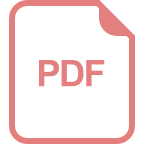