js canvas物体从左到右的运动 频繁调用canvasApi优化
时间: 2024-12-05 15:13:07 浏览: 6
在JavaScript中使用Canvas API进行物体从左到右的运动时,频繁调用Canvas API可能会导致性能问题。为了优化这种情况,可以采取以下几种方法:
1. **减少重绘区域**:只重绘需要更新的部分,而不是整个Canvas。可以使用`clearRect`方法清除需要更新的区域,然后重新绘制物体。
2. **使用离屏Canvas**:将静态部分绘制到一个离屏Canvas上,然后在主Canvas上绘制这个离屏Canvas。这样可以减少每次重绘时的计算量。
3. **使用`requestAnimationFrame`**:相比于`setInterval`或`setTimeout`,`requestAnimationFrame`更高效,因为它会在浏览器下一次重绘之前调用回调函数,从而优化动画性能。
4. **批量绘制**:尽量减少绘制调用的次数,将多个绘制操作合并成一个。
以下是一个示例代码,展示了如何优化Canvas上的物体运动:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Canvas Animation Optimization</title>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="myCanvas" width="800" height="400"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
let x = 0;
const speed = 2;
// 离屏Canvas
const offCanvas = document.createElement('canvas');
offCanvas.width = canvas.width;
offCanvas.height = canvas.height;
const offCtx = offCanvas.getContext('2d');
// 绘制静态背景
offCtx.fillStyle = 'lightblue';
offCtx.fillRect(0, 0, canvas.width, canvas.height);
// 绘制物体
function draw() {
// 清除需要更新的区域
ctx.clearRect(x, 0, 50, canvas.height);
// 绘制物体
ctx.fillStyle = 'red';
ctx.fillRect(x, 175, 50, 50);
// 更新位置
x += speed;
// 如果物体超出屏幕,重置位置
if (x > canvas.width) {
x = -50;
}
// 请求下一帧
requestAnimationFrame(draw);
}
// 开始动画
draw();
</script>
</body>
</html>
```
在这个示例中,我们使用了离屏Canvas来绘制静态背景,并使用`requestAnimationFrame`来优化动画性能。
阅读全文
相关推荐


















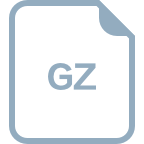