JavaScript ajax表单验证代码
时间: 2024-10-20 17:02:39 浏览: 15
在JavaScript中,AJAX(Asynchronous JavaScript and XML)用于创建异步的HTTP请求,无需刷新整个页面即可与服务器交换数据。下面是一个简单的表单验证的AJAX示例,当用户提交表单前,会检查输入是否有效:
```javascript
// 获取表单元素
const form = document.getElementById('myForm');
const nameInput = document.getElementById('name');
const emailInput = document.getElementById('email');
// 创建一个预设的错误消息容器
const errorMessage = document.getElementById('error-message');
// 验证函数
function validateForm(event) {
event.preventDefault(); // 阻止表单默认提交
const nameValue = nameInput.value.trim();
const emailValue = emailInput.value.trim();
// 清空错误信息
errorMessage.textContent = '';
if (nameValue === '') {
errorMessage.textContent = '名字不能为空';
} else if (!isValidEmail(emailValue)) {
errorMessage.textContent = '请输入有效的电子邮件地址';
} else {
// 如果所有验证都通过,使用AJAX发送数据
sendAjaxRequest(nameValue, emailValue);
}
}
// 检查电子邮件地址是否有效(这里只是一个基本的正则表达式)
function isValidEmail(email) {
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(email);
}
// AJAX请求示例(假设你已经有了一个sendAjaxRequest函数)
function sendAjaxRequest(name, email) {
fetch('/api/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ name, email })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
}
// 当表单提交事件触发时,执行验证函数
form.addEventListener('submit', validateForm);
```
在这个例子中,`validateForm`函数会在表单提交时执行,如果验证失败,它会显示错误信息并阻止表单提交。如果验证成功,就会通过`sendAjaxRequest`函数发送数据到服务器。
阅读全文
相关推荐
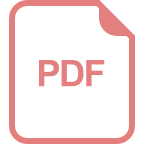
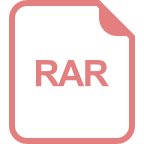
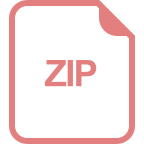
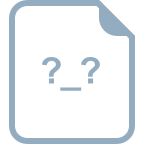
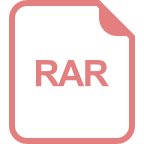
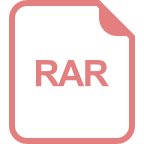
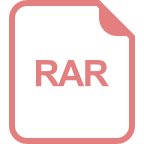
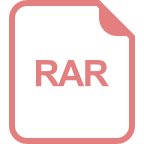
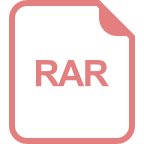
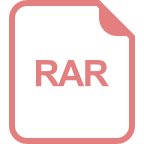
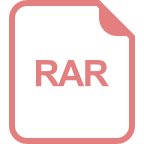
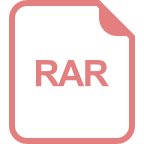
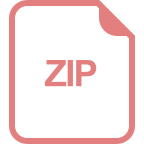
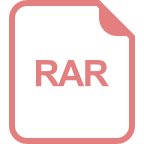
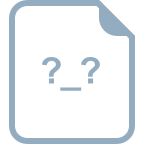
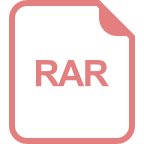
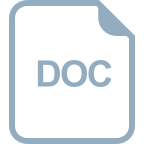
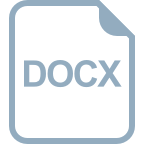