双目位运算计算函数C语言
时间: 2023-11-11 07:14:13 浏览: 159
双目位运算符是C语言中的一种运算符,它可以对两个整数进行位运算操作。常见的双目位运算符包括按位与(&)、按位或(|)、按位异或(^)等。
以下是三种常见的双目位运算计算函数的实现:
1. 按位与(&)运算计算函数:
```c
int bitwiseAnd(int a, int b) {
return a & b;
}
```
2. 按位或(|)运算计算函数:
```c
int bitwiseOr(int a, int b) {
return a | b;
}
```
3. 按位异或(^)运算计算函数:
```c
int bitwiseXor(int a, int b) {
return a ^ b;
}
```
以上函数中,a和b是要进行运算的两个整数。函数返回值是运算结果。这些函数可以在程序中直接调用,例如:
```c
int a = 15; // 二进制表示为 1111
int b = 5; // 二进制表示为 0101
int c = bitwiseAnd(a, b); // c的值为 5(二进制表示为 0101)
int d = bitwiseOr(a, b); // d的值为 15(二进制表示为 1111)
int e = bitwiseXor(a, b); // e的值为 10(二进制表示为 1010)
```
相关问题
用从c语言合理运用栈,按照教材中的运算优先级,编程实现任意中缀算术表达式(可以只包含’+’、’-‘、’*’、’/’等双目运算符、小括号和结束符)的求值运算。
要实现任意中缀算术表达式的求值运算,可以使用栈来存储运算符和操作数。按照运算优先级,遍历中缀表达式的每个字符,根据字符的类型进行相应的处理。当遇到操作数时,将其压入操作数栈中;当遇到运算符时,将其与运算符栈中的栈顶元素进行比较,如果当前运算符的优先级较高,则将其压入运算符栈中;如果当前运算符的优先级较低,则从运算符栈中弹出栈顶运算符,并从操作数栈中弹出两个操作数,进行相应的计算,将结果压入操作数栈中;当遇到小括号时,将小括号内的表达式视为一个整体,按照相同的逻辑进行处理。
示例代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
// 定义栈结构
typedef struct {
int top; // 栈顶指针
int capacity; // 栈的容量
int* array; // 栈数组
} Stack;
// 创建栈
Stack* createStack(int capacity) {
Stack* stack = (Stack*)malloc(sizeof(Stack));
stack->top = -1;
stack->capacity = capacity;
stack->array = (int*)malloc(stack->capacity * sizeof(int));
return stack;
}
// 判断栈是否为空
int isEmpty(Stack* stack) {
return stack->top == -1;
}
// 判断栈是否满
int isFull(Stack* stack) {
return stack->top == stack->capacity - 1;
}
// 入栈操作
void push(Stack* stack, int item) {
if (isFull(stack)) {
printf("Stack is full.\n");
return;
}
stack->array[++stack->top] = item;
}
// 出栈操作
int pop(Stack* stack) {
if (isEmpty(stack)) {
printf("Stack is empty.\n");
return -1;
}
return stack->array[stack->top--];
}
// 获取栈顶元素
int peek(Stack* stack) {
if (isEmpty(stack)) {
printf("Stack is empty.\n");
return -1;
}
return stack->array[stack->top];
}
// 判断运算符的优先级
int precedence(char op) {
if (op == '+' || op == '-')
return 1;
if (op == '*' || op == '/')
return 2;
return 0;
}
// 执行运算
int evaluate(int a, int b, char op) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
default:
return -1;
}
}
// 中缀表达式求值函数
int evaluateInfixExpression(char* expression) {
Stack* operandStack = createStack(100);
Stack* operatorStack = createStack(100);
int i = 0;
while (expression[i] != '\0') {
if (isdigit(expression[i])) { // 操作数
int operand = 0;
while (isdigit(expression[i])) {
operand = operand * 10 + (expression[i] - '0');
i++;
}
push(operandStack, operand);
} else if (expression[i] == '(') { // 左括号
push(operatorStack, expression[i]);
i++;
} else if (expression[i] == ')') { // 右括号
while (peek(operatorStack) != '(') {
char op = pop(operatorStack);
int b = pop(operandStack);
int a = pop(operandStack);
int result = evaluate(a, b, op);
push(operandStack, result);
}
pop(operatorStack); // 弹出左括号
i++;
} else { // 运算符
while (!isEmpty(operatorStack) && precedence(expression[i]) <= precedence(peek(operatorStack))) {
char op = pop(operatorStack);
int b = pop(operandStack);
int a = pop(operandStack);
int result = evaluate(a, b, op);
push(operandStack, result);
}
push(operatorStack, expression[i]);
i++;
}
}
while (!isEmpty(operatorStack)) {
char op = pop(operatorStack);
int b = pop(operandStack);
int a = pop(operandStack);
int result = evaluate(a, b, op);
push(operandStack, result);
}
int finalResult = pop(operandStack);
free(operandStack);
free(operatorStack);
return finalResult;
}
int main() {
char expression[100];
printf("请输入中缀表达式:");
scanf("%s", expression);
int result = evaluateInfixExpression(expression);
printf("表达式的求值结果为:%d\n", result);
return 0;
}
```
阅读全文
相关推荐
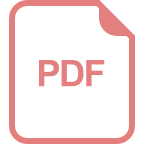
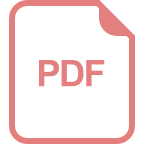
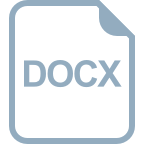
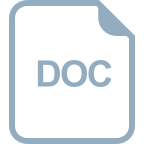
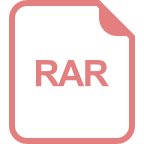
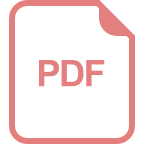
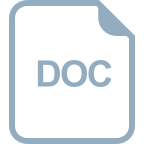
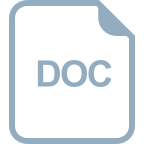
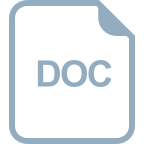
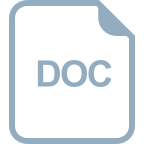
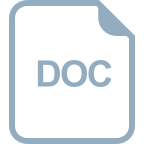
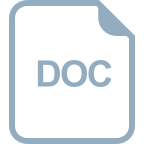
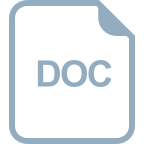
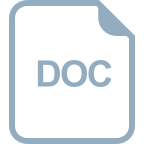
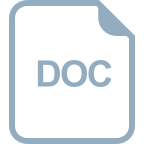
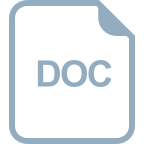
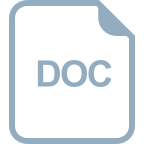