使用java开发根据模板自动生成行为树xml文件
时间: 2024-03-01 18:05:24 浏览: 106
好的,使用Java实现自动生成行为树xml文件的过程可以分为以下几个步骤:
1. 定义行为树的节点类型和基本结构。可以使用枚举类型来定义节点类型,使用类来定义节点信息,包括节点名称、节点类型、节点参数等。
```java
public enum NodeType {
Selector, Sequence, Condition, Action
}
public class Node {
private String name;
private NodeType type;
private Map<String, Object> params;
private List<Node> children;
// getters and setters
}
```
2. 编写行为树的生成器。可以使用递归的方式,遍历所有节点并根据节点类型生成对应的xml元素。
```java
public class BehaviorTreeGenerator {
public static Element generate(Node node) {
Element element;
switch (node.getType()) {
case Selector:
element = new Element("Selector");
break;
case Sequence:
element = new Element("Sequence");
break;
case Condition:
element = new Element("Condition");
break;
case Action:
element = new Element("Action");
break;
default:
throw new RuntimeException("Invalid node type: " + node.getType());
}
element.setAttribute("name", node.getName());
// add parameters
for (Map.Entry<String, Object> entry : node.getParams().entrySet()) {
element.setAttribute(entry.getKey(), entry.getValue().toString());
}
// add children
for (Node child : node.getChildren()) {
element.addContent(generate(child));
}
return element;
}
}
```
3. 使用生成器来生成xml文件。可以使用JDOM库来创建xml文件和元素,并将生成的节点树添加到xml文件中。
```java
public class Main {
public static void main(String[] args) {
Node root = new Node("root", NodeType.Selector);
Node condition = new Node("condition", NodeType.Condition);
condition.getParams().put("param1", "value1");
Node action = new Node("action", NodeType.Action);
action.getParams().put("param2", "value2");
root.getChildren().add(condition);
root.getChildren().add(action);
Element rootElement = new Element("BehaviorTree");
rootElement.addContent(BehaviorTreeGenerator.generate(root));
Document doc = new Document(rootElement);
XMLOutputter outputter = new XMLOutputter(Format.getPrettyFormat());
try {
outputter.output(doc, new FileOutputStream("behavior_tree.xml"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这样就可以根据模板自动生成行为树xml文件了。需要注意的是,生成器的实现和xml文件的格式可以根据具体需求进行修改和扩展。
阅读全文
相关推荐
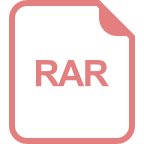
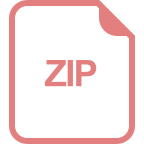
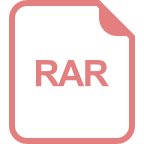
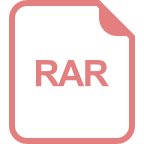
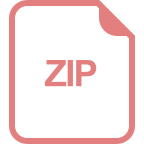
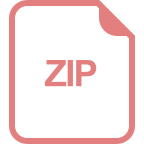
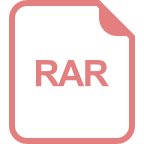
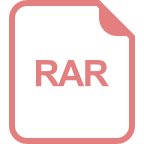
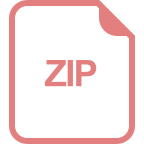
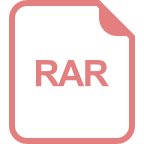
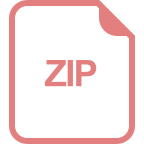
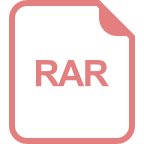
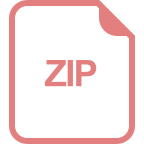
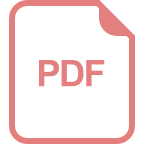
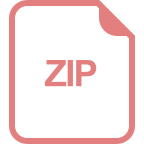