用python实现随机森林分类的10折交叉验证,并且输出准确率,召回率和F1值
时间: 2023-06-18 13:08:46 浏览: 232
下面是一个用Python实现随机森林分类的10折交叉验证,并输出准确率,召回率和F1值的示例代码:
```python
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, recall_score, f1_score
from sklearn.model_selection import KFold
import numpy as np
# 假设数据集为X和y
X = np.array([[1, 2], [3, 4], [5, 6], [7, 8]])
y = np.array([0, 1, 0, 1])
# 设置随机森林分类器参数
n_estimators = 100
max_depth = 5
random_state = 42
# 初始化 10 折交叉验证器
kf = KFold(n_splits=10, shuffle=True, random_state=42)
# 初始化评估指标列表
accuracy_scores, recall_scores, f1_scores = [], [], []
# 开始 10 折交叉验证
for train_index, test_index in kf.split(X):
# 划分训练和测试集
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
# 训练随机森林分类器
clf = RandomForestClassifier(n_estimators=n_estimators, max_depth=max_depth, random_state=random_state)
clf.fit(X_train, y_train)
# 预测测试集
y_pred = clf.predict(X_test)
# 计算准确率、召回率和 F1 值
accuracy_scores.append(accuracy_score(y_test, y_pred))
recall_scores.append(recall_score(y_test, y_pred))
f1_scores.append(f1_score(y_test, y_pred))
# 输出平均值
print('Average accuracy: {:.2f}'.format(np.mean(accuracy_scores)))
print('Average recall: {:.2f}'.format(np.mean(recall_scores)))
print('Average F1 score: {:.2f}'.format(np.mean(f1_scores)))
```
其中,`X`是数据集的特征矩阵,`y`是数据集的标签向量。随机森林分类器的参数包括`n_estimators`(决策树数量)、`max_depth`(每棵决策树的最大深度)和`random_state`(随机种子)。交叉验证器使用`KFold`,将数据集划分为10个子集,每次取其中一个子集作为测试集,其余作为训练集。在每次交叉验证中,训练随机森林分类器并预测测试集,计算准确率、召回率和F1值。最后,输出平均值。
阅读全文
相关推荐
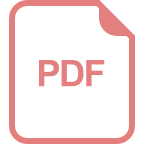
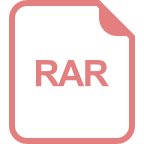
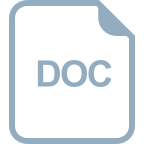

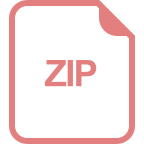
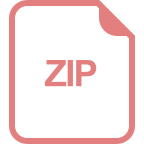
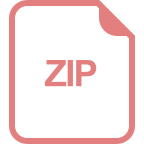
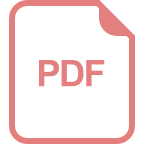
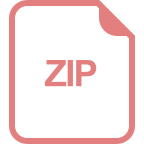
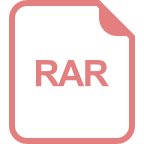
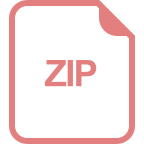
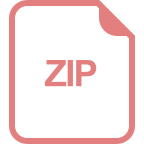
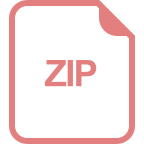
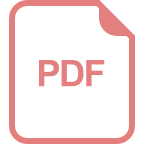
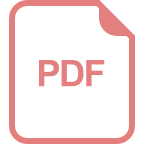