data1.query('Country=="Netherlands" ')怎么得到每月的Price
时间: 2024-03-18 21:39:56 浏览: 19
假设`data1`是一个包含Country和Price列的数据框,你想要得到每个月Netherlands的Price,可以使用下面的代码:
```
# 将日期转换为月份
data1['Month'] = pd.to_datetime(data1['Date']).dt.to_period('M')
# 筛选出Netherlands的数据
netherlands_data = data1.query('Country == "Netherlands"')
# 以月份为分组键,计算每月的Price的平均值
monthly_price = netherlands_data.groupby('Month')['Price'].mean()
```
这个代码中,首先将日期转换为月份,然后筛选出Netherlands的数据,最后以月份为分组键,计算每月的Price的平均值。最终的结果是一个Series对象,其中索引是月份,值是该月的平均Price。
相关问题
net_data=data1.query('Country=="Netherlands" and Quantity>0').groupby(data1['InvoiceDate'].dt.strftime('%Y-%m'))['Price'].sum().reset_index() eire_data=data1.query('Country=="EIRE" and Quantity>0').groupby(data1['InvoiceDate'].dt.strftime('%Y-%m'))['Price'].sum().reset_index()怎么生成柱状图对比
要生成柱状图对比,您可以使用matplotlib库来绘制图表。以下是一个示例代码:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取数据
data1 = pd.read_csv('your_file_path.csv')
# 将InvoiceDate列转换为日期类型
data1['InvoiceDate'] = pd.to_datetime(data1['InvoiceDate'])
# 提取Netherlands和EIRE的销售数据
net_data = data1.query('Country=="Netherlands" and Quantity>0').groupby(data1['InvoiceDate'].dt.strftime('%Y-%m'))['Price'].sum().reset_index()
eire_data = data1.query('Country=="EIRE" and Quantity>0').groupby(data1['InvoiceDate'].dt.strftime('%Y-%m'))['Price'].sum().reset_index()
# 绘制柱状图
plt.bar(net_data['InvoiceDate'], net_data['Price'], label='Netherlands')
plt.bar(eire_data['InvoiceDate'], eire_data['Price'], label='EIRE')
# 添加图表标题和标签
plt.title('Sales Comparison between Netherlands and EIRE')
plt.xlabel('Year-Month')
plt.ylabel('Sales')
plt.legend()
# 显示图表
plt.show()
```
在上面的代码中,您需要将'your_file_path.csv'替换为您的数据文件路径。然后,使用`pd.read_csv()`函数读取数据,并使用`pd.to_datetime()`方法将InvoiceDate列转换为日期类型。接下来,使用`.query()`方法和`.groupby()`方法提取Netherlands和EIRE的销售数据,并使用`.reset_index()`方法重置索引以便于绘制图表。然后,使用`plt.bar()`函数绘制柱状图,并使用`plt.title()`、`plt.xlabel()`、`plt.ylabel()`和`plt.legend()`函数添加图表标题、标签和图例。最后,使用`plt.show()`函数显示图表。
请注意,如果您的数据文件中没有Netherlands和EIRE的数据,您需要根据实际情况修改代码中的查询条件。另外,您可以根据需要修改图表的样式和布局。
from pyecharts import options as opts from pyecharts.charts import Bar from pyecharts.globals import ThemeType net_data=data1.query('Country=="Netherlands" and Quantity>0').groupby(data1['InvoiceDate'] .dt.strftime('%Y-%m'))['Price'].sum().reset_index() eire_data=data1.query('Country=="EIRE" and Quantity>0').groupby(data1['InvoiceDate'] .dt.strftime('%Y-%m'))['Price'].sum().reset_index() bar = ( Bar(init_opts=opts.InitOpts(width='1000px',height='600px')) .add_xaxis(list(net_data['InvoiceDate'])) .add_yaxis("荷兰", list(net_data['Price'])) .add_yaxis("爱尔兰", list(eire_data['Price'])) .set_global_opts(title_opts=opts.TitleOpts(title="荷兰与爱尔兰每月销售情况对比"),toolbox_opts=opts.ToolboxOpts()) .set_series_opts(markline_opts=opts.MarkLineOpts(data=[opts.MarkLineItem(type_='average',name='平均值')])) .set_series_opts(markpoint_opts=opts.MarkPointOpts(data=[opts.MarkPointItem(type_='max',name='最大值') ,opts.MarkPointItem(type_='min',name='最小值')])) ) bar.render_notebook()加上注释
好的,这段代码使用 pyecharts 库绘制了荷兰与爱尔兰每月销售情况的对比柱状图。
```python
# 从数据集中选出荷兰每月销售额数据
net_data = data1.query('Country=="Netherlands" and Quantity>0').groupby(data1['InvoiceDate'].dt.strftime('%Y-%m'))['Price'].sum().reset_index()
# 从数据集中选出爱尔兰每月销售额数据
eire_data = data1.query('Country=="EIRE" and Quantity>0').groupby(data1['InvoiceDate'].dt.strftime('%Y-%m'))['Price'].sum().reset_index()
# 创建柱状图,设置宽度和高度
bar = (
Bar(init_opts=opts.InitOpts(width='1000px',height='600px'))
# 添加x轴数据和两个y轴数据
.add_xaxis(list(net_data['InvoiceDate']))
.add_yaxis("荷兰", list(net_data['Price']))
.add_yaxis("爱尔兰", list(eire_data['Price']))
# 设置全局选项,包括标题和工具箱
.set_global_opts(title_opts=opts.TitleOpts(title="荷兰与爱尔兰每月销售情况对比"),toolbox_opts=opts.ToolboxOpts())
# 设置系列选项,包括标记线和标记点
.set_series_opts(markline_opts=opts.MarkLineOpts(data=[opts.MarkLineItem(type_='average',name='平均值')]))
.set_series_opts(markpoint_opts=opts.MarkPointOpts(data=[opts.MarkPointItem(type_='max',name='最大值')
,opts.MarkPointItem(type_='min',name='最小值')]))
)
# 在notebook中渲染柱状图
bar.render_notebook()
```
该代码通过对数据集的筛选和分组,获得荷兰和爱尔兰每月的销售额数据,并使用 pyecharts 库创建柱状图。图表中展示了荷兰和爱尔兰每月的销售额情况,并标出了平均值、最大值和最小值。
相关推荐
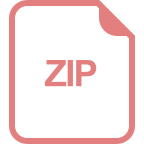
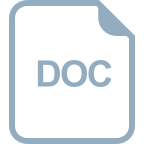












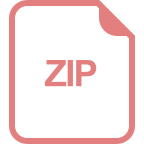