th:action="delete"><a th:href="@{todelete)">刚除</a> 解释
时间: 2023-11-01 10:08:09 浏览: 27
这段代码是一个使用了Thymeleaf模板引擎的HTML片段,其中th:action="delete"表示在表单提交时将请求发送到一个名为"delete"的控制器,而<a th:href="@{todelete)}">标签是一个超链接,当用户点击它时,它也会将请求发送到"delete"控制器。这个控制器将负责处理删除操作。
相关问题
<body> <form action="QueryServlet" method="post"> 请输入用户名:<input type="text" name="userName"/> <input type="submit" value="查询"/> <a href="GetAllUsersServlet">显示全部</a> </form> <table border=1> <tr><th>用户名</th><th>密码</th><th>积分</th><th>注册时间</th></tr> <c:forEach items="${users }" var="item"> <tr> <td>${item.userName }</td> <td>${item.password }</td> <td>${item.integral }</td> <td>${item.registerTime }</td> <td><a href="">删除</a></td> </tr> </c:forEach> </table> </body>tomcat 删除一条记录怎么写
要实现删除一条记录,需要完成以下步骤:
1. 在表格中为每行添加一个“删除”链接,可以通过在<tr>标签内添加一个<a>标签实现,例如:
```
<td><a href="DeleteServlet?id=${item.id}">删除</a></td>
```
其中,`${item.id}`表示当前行的记录ID,将其作为参数传递给DeleteServlet。
2. 在DeleteServlet中,获取从前端传递过来的ID参数,使用该ID在数据库中执行删除操作。具体代码如下:
```
String id = request.getParameter("id");
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DBUtils.getConnection();
String sql = "DELETE FROM user WHERE id=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtils.close(conn, pstmt, null);
}
```
其中,DBUtils是一个封装了JDBC操作的工具类,getConnection()用于获取数据库连接,close()用于关闭连接和释放资源。执行删除操作时,使用预编译的SQL语句,使用参数占位符“?”代替ID的值,防止SQL注入攻击。最后,使用executeUpdate()方法执行SQL语句,删除对应的记录。
3. 在web.xml文件中配置DeleteServlet的映射关系,例如:
```
<servlet>
<servlet-name>DeleteServlet</servlet-name>
<servlet-class>com.example.DeleteServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>DeleteServlet</servlet-name>
<url-pattern>/DeleteServlet</url-pattern>
</servlet-mapping>
```
这样,当用户点击“删除”链接时,就会向DeleteServlet发送一个HTTP请求,DeleteServlet会根据请求参数执行删除操作。
帮我看看下面的代码为啥有问题,并纠正:<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>学生信息</title> <script type="text/javascript"> function studentDelete(studentId) { if(confirm("你确定要删除这个学生信息吗?")){ windows.location="deletestudent?userId="+studentId; } } </script> </head> <body> <table border=1> <tr> <th>学号</th> <th>姓名</th> <th>性别</th> <th>出生年龄</th> <th>入学时间</th> <th>学院</th> <th>操作</th> </tr> <c:forEach items="${stuList} " var="stu"> <tr> <td>${stu.userId}</td> <td>${stu.userName}</td> <td>${stu.sex}</td> <td>${stu.birthYear}</td> <td>${stu.grad}</td> <td>${stu.collegeName}</td> <td> <button type="button" onclick="Location='AdminServlet?action=edit$userId=${stu.userId}'">修改</button> <button type="button" onclick="studentDeLete(${stu.userId})">删除</button> </td> </tr> </c:forEach> </table> </body> </html>
这段代码有几个问题需要修正:
1. 在 `<script>` 标签中,`windows.location` 应该是 `window.location`,即将 `windows` 改为 `window`。
2. 在 `<c:forEach>` 标签中,`${stuList}` 后面有一个多余的空格,需要删除。
3. 在 `<button>` 标签中,`onclick` 属性中的 `Location` 应该是小写的 `location`,即将 `Location` 改为 `location`。
4. 在第二个 `<button>` 标签中,`onclick` 属性中的 `studentDeLete` 应该是 `studentDelete`,即将 `studentDeLete` 改为 `studentDelete`。
修正后的代码如下所示:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生信息</title>
<script type="text/javascript">
function studentDelete(studentId) {
if(confirm("你确定要删除这个学生信息吗?")){
window.location="deletestudent?userId="+studentId;
}
}
</script>
</head>
<body>
<table border=1>
<tr>
<th>学号</th>
<th>姓名</th>
<th>性别</th>
<th>出生年龄</th>
<th>入学时间</th>
<th>学院</th>
<th>操作</th>
</tr>
<c:forEach items="${stuList}" var="stu">
<tr>
<td>${stu.userId}</td>
<td>${stu.userName}</td>
<td>${stu.sex}</td>
<td>${stu.birthYear}</td>
<td>${stu.grad}</td>
<td>${stu.collegeName}</td>
<td>
<button type="button" onclick="location='AdminServlet?action=edit$userId=${stu.userId}'">修改</button>
<button type="button" onclick="studentDelete(${stu.userId})">删除</button>
</td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
请注意检查其他部分的代码,以确保没有其他错误。
相关推荐
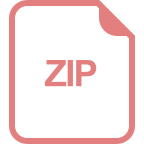
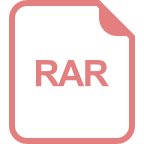
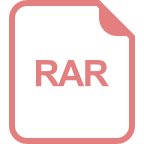

{% extends "admin/base.html" %} {% block content %} 药品列表 ID 药品名称 操作 {% for drug in drugs %} {{ drug.id }} {{ drug.drug_name }} 编辑 <form action="{% url 'admin:drug_db_drug_list_delete' drug.id %}" method="post" class="d-inline"> {% csrf_token %} <button class="btn btn-danger btn-sm">删除</button> </form> {% empty %} 暂无药品 {% endfor %} 添加新药品 药品相互作用 ID 药品1 药品2 相互作用 操作 {% for interaction in interactions %} {{ interaction.id }} {{ interaction.drug1 }} {{ interaction.drug2 }} {{ interaction.interaction }} 编辑 <form action="{% url 'admin:drug_db_drug_interaction_delete' interaction.id %}" method="post" class="d-inline"> {% csrf_token %} <button class="btn btn-danger btn-sm">删除</button> </form> {% empty %} 暂无药品相互作用 {% endfor %} 添加新药品相互作用 {% endblock %} 需要定义那些视图和建那些文件
