数据结构中编写一个实现串的置换操作Replace(&S, T, V)的算法,用C语言编写
时间: 2024-10-23 07:20:03 浏览: 22
在C语言中,你可以使用两个指针分别指向字符串S和T,然后遍历字符串S,对于每个字符,如果它等于T中的当前字符,就将其替换为V。这里是一个简单的实现:
```c
#include <stdio.h>
#include <string.h>
// 函数声明
void replace(char* S, char* T, char V);
int main() {
char str1[100], str2[100], replacement;
printf("Enter the string S: ");
fgets(str1, sizeof(str1), stdin); // 注意去掉fgets末尾的换行符
str1[strlen(str1)-1] = '\0'; // 修复fgets留下的空字符
printf("Enter the pattern to search (T): ");
fgets(str2, sizeof(str2), stdin);
str2[strlen(str2)-1] = '\0';
printf("Enter the character to replace (V): ");
scanf("%c", &replacement);
replace(str1, str2, replacement);
printf("After replacing: %s\n", str1);
return 0;
}
// 替换函数实现
void replace(char* S, char* T, char V) {
int i = 0, j = 0; // 指向S和T的指针
while (S[i]) { // 遍历字符串S直到遇到'\0'
if (S[i] == T[j]) {
S[i] = V; // 如果匹配,替换S[i]
j++;
}
i++; // 继续处理下一个字符
}
// 如果T比S长,将剩余的部分恢复原样
for (; j < strlen(T); j++) {
S[i++] = T[j];
}
}
```
这个程序首先让用户输入原始字符串S、搜索模式T和替换字符V,然后`replace`函数遍历S并对找到的匹配项进行替换。
阅读全文
相关推荐
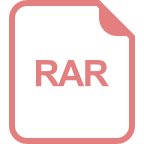
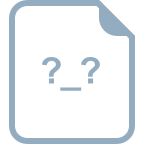
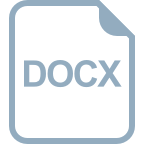















