react 如何写一个异步
时间: 2024-05-13 20:20:46 浏览: 10
React 是一个视图层框架,不直接涉及异步操作。但是,我们可以在 React 中使用异步操作,例如在组件生命周期方法中发起异步请求或使用异步操作来更新组件状态。
以下是一些常见的异步操作示例:
1. 在 componentDidMount() 生命周期方法中发起异步请求:
```
class MyComponent extends React.Component {
state = {
data: null
}
async componentDidMount() {
const response = await fetch('https://api.example.com/data')
const data = await response.json()
this.setState({ data })
}
render() {
return <div>{this.state.data ? this.state.data.message : 'Loading...'}</div>
}
}
```
2. 使用 Promise 和 async/await 更新组件状态:
```
class MyComponent extends React.Component {
state = {
loading: true,
error: null,
data: null
}
async fetchData() {
this.setState({ loading: true })
try {
const response = await fetch('https://api.example.com/data')
const data = await response.json()
this.setState({ loading: false, data })
} catch (error) {
this.setState({ loading: false, error })
}
}
componentDidMount() {
this.fetchData()
}
render() {
if (this.state.loading) {
return <div>Loading...</div>
}
if (this.state.error) {
return <div>Error: {this.state.error.message}</div>
}
return <div>{this.state.data.message}</div>
}
}
```
3. 使用 Promise 和 then() 更新组件状态:
```
class MyComponent extends React.Component {
state = {
loading: true,
error: null,
data: null
}
componentDidMount() {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => this.setState({ loading: false, data }))
.catch(error => this.setState({ loading: false, error }))
}
render() {
if (this.state.loading) {
return <div>Loading...</div>
}
if (this.state.error) {
return <div>Error: {this.state.error.message}</div>
}
return <div>{this.state.data.message}</div>
}
}
```
相关推荐
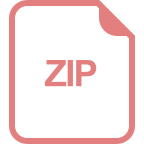
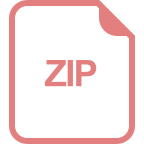














