以#include <iostream> #include <windows.h> #include <iomanip> #define N 1000 using namespace std; struct person { int age char function[12] char gender[10] }; struct staff { int number; char name[12] person per;开头写一篇关于员工信息管理的小系统
时间: 2024-01-05 10:03:36 浏览: 64
员工信息管理系统
本系统旨在帮助企业或机构管理员工信息,包括员工基本信息、工作信息等。本系统使用 C++ 语言编写,采用结构体和文件存储方式实现员工信息的管理和查询。
系统功能
1. 添加员工信息
2. 查询员工信息
3. 修改员工信息
4. 删除员工信息
5. 显示所有员工信息
6. 退出系统
系统实现
1. 添加员工信息
当用户选择添加员工信息时,系统会要求用户输入员工的基本信息,包括员工编号、姓名、年龄、性别、职务等。在输入完毕后,系统将员工信息保存到文件中,以便后续查询和修改。
2. 查询员工信息
当用户选择查询员工信息时,系统会要求用户输入员工编号或姓名,并根据用户的输入在文件中查找员工信息。如果找到了对应的员工信息,系统将显示该员工的基本信息、工作信息等;否则,系统将提示用户未找到对应的员工信息。
3. 修改员工信息
当用户选择修改员工信息时,系统会要求用户输入员工编号或姓名,并根据用户的输入在文件中查找员工信息。如果找到了对应的员工信息,系统将允许用户修改员工的基本信息、工作信息等;否则,系统将提示用户未找到对应的员工信息。
4. 删除员工信息
当用户选择删除员工信息时,系统会要求用户输入员工编号或姓名,并根据用户的输入在文件中查找员工信息。如果找到了对应的员工信息,系统将提示用户确认是否删除该员工信息;否则,系统将提示用户未找到对应的员工信息。
5. 显示所有员工信息
当用户选择显示所有员工信息时,系统将从文件中读取所有员工信息,并将其显示在屏幕上,包括员工的基本信息、工作信息等。
6. 退出系统
当用户选择退出系统时,系统将保存所有员工信息,并退出程序。
源码实现
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <cstdlib>
#include <windows.h>
#include <iomanip>
using namespace std;
#define N 1000
struct Person {
int age;
char function[12];
char gender[10];
};
struct Staff {
int number;
char name[12];
Person per;
};
void add_staff(Staff staffs[], int& count) {
cout << "请输入员工编号:";
cin >> staffs[count].number;
cout << "请输入员工姓名:";
cin >> staffs[count].name;
cout << "请输入员工年龄:";
cin >> staffs[count].per.age;
cout << "请输入员工性别:";
cin >> staffs[count].per.gender;
cout << "请输入员工职务:";
cin >> staffs[count].per.function;
count++;
cout << "添加成功!" << endl;
}
void search_staff(Staff staffs[], int count) {
int choice;
string name;
int number;
cout << "请选择查询方式(1.按编号查询,2.按姓名查询):";
cin >> choice;
if (choice == 1) {
cout << "请输入员工编号:";
cin >> number;
for (int i = 0; i < count; i++) {
if (staffs[i].number == number) {
cout << "员工编号:" << staffs[i].number << endl;
cout << "员工姓名:" << staffs[i].name << endl;
cout << "员工年龄:" << staffs[i].per.age << endl;
cout << "员工性别:" << staffs[i].per.gender << endl;
cout << "员工职务:" << staffs[i].per.function << endl;
return;
}
}
}
else if (choice == 2) {
cout << "请输入员工姓名:";
cin >> name;
for (int i = 0; i < count; i++) {
if (strcmp(staffs[i].name, name.c_str()) == 0) {
cout << "员工编号:" << staffs[i].number << endl;
cout << "员工姓名:" << staffs[i].name << endl;
cout << "员工年龄:" << staffs[i].per.age << endl;
cout << "员工性别:" << staffs[i].per.gender << endl;
cout << "员工职务:" << staffs[i].per.function << endl;
return;
}
}
}
cout << "未找到对应的员工信息!" << endl;
}
void modify_staff(Staff staffs[], int count) {
int choice;
string name;
int number;
cout << "请选择修改方式(1.按编号修改,2.按姓名修改):";
cin >> choice;
if (choice == 1) {
cout << "请输入员工编号:";
cin >> number;
for (int i = 0; i < count; i++) {
if (staffs[i].number == number) {
cout << "请输入员工姓名:";
cin >> staffs[i].name;
cout << "请输入员工年龄:";
cin >> staffs[i].per.age;
cout << "请输入员工性别:";
cin >> staffs[i].per.gender;
cout << "请输入员工职务:";
cin >> staffs[i].per.function;
cout << "修改成功!" << endl;
return;
}
}
}
else if (choice == 2) {
cout << "请输入员工姓名:";
cin >> name;
for (int i = 0; i < count; i++) {
if (strcmp(staffs[i].name, name.c_str()) == 0) {
cout << "请输入员工编号:";
cin >> staffs[i].number;
cout << "请输入员工年龄:";
cin >> staffs[i].per.age;
cout << "请输入员工性别:";
cin >> staffs[i].per.gender;
cout << "请输入员工职务:";
cin >> staffs[i].per.function;
cout << "修改成功!" << endl;
return;
}
}
}
cout << "未找到对应的员工信息!" << endl;
}
void delete_staff(Staff staffs[], int& count) {
int choice;
string name;
int number;
cout << "请选择删除方式(1.按编号删除,2.按姓名删除):";
cin >> choice;
if (choice == 1) {
cout << "请输入员工编号:";
cin >> number;
for (int i = 0; i < count; i++) {
if (staffs[i].number == number) {
for (int j = i; j < count - 1; j++) {
staffs[j] = staffs[j + 1];
}
count--;
cout << "删除成功!" << endl;
return;
}
}
}
else if (choice == 2) {
cout << "请输入员工姓名:";
cin >> name;
for (int i = 0; i < count; i++) {
if (strcmp(staffs[i].name, name.c_str()) == 0) {
for (int j = i; j < count - 1; j++) {
staffs[j] = staffs[j + 1];
}
count--;
cout << "删除成功!" << endl;
return;
}
}
}
cout << "未找到对应的员工信息!" << endl;
}
void display_staff(Staff staffs[], int count) {
cout << "员工编号\t员工姓名\t员工年龄\t员工性别\t员工职务" << endl;
for (int i = 0; i < count; i++) {
cout << staffs[i].number << "\t\t" << staffs[i].name << "\t\t" << staffs[i].per.age << "\t\t" << staffs[i].per.gender << "\t\t" << staffs[i].per.function << endl;
}
}
int main() {
Staff staffs[N];
int count = 0;
int choice;
ifstream infile("staffs.txt");
if (infile.is_open()) {
while (!infile.eof() && count < N) {
infile >> staffs[count].number;
infile >> staffs[count].name;
infile >> staffs[count].per.age;
infile >> staffs[count].per.gender;
infile >> staffs[count].per.function;
count++;
}
infile.close();
}
while (true) {
cout << "-------------------员工信息管理系统-------------------" << endl;
cout << "1.添加员工信息" << endl;
cout << "2.查询员工信息" << endl;
cout << "3.修改员工信息" << endl;
cout << "4.删除员工信息" << endl;
cout << "5.显示所有员工信息" << endl;
cout << "6.退出系统" << endl;
cout << "请选择操作(1-6):";
cin >> choice;
switch (choice) {
case 1:
add_staff(staffs, count);
break;
case 2:
search_staff(staffs, count);
break;
case 3:
modify_staff(staffs, count);
break;
case 4:
delete_staff(staffs, count);
break;
case 5:
display_staff(staffs, count);
break;
case 6:
ofstream outfile("staffs.txt");
for (int i = 0; i < count; i++) {
outfile << staffs[i].number << " ";
outfile << staffs[i].name << " ";
outfile << staffs[i].per.age << " ";
outfile << staffs[i].per.gender << " ";
outfile << staffs[i].per.function << endl;
}
outfile.close();
cout << "感谢使用员工信息管理系统,再见!" << endl;
Sleep(2000);
exit(0);
default:
cout << "无效操作,请重新选择!" << endl;
break;
}
system("pause");
system("cls");
}
return 0;
}
```
系统说明
本系统使用了文件存储方式,将员工信息保存到文本文件中。在程序运行时,系统会从文件中读取已有的员工信息,并将修改后的员工信息保存到文件中。由于本系统仅为演示程序,没有进行输入检查和异常处理,实际使用时需要进行相应的改进。
阅读全文
相关推荐










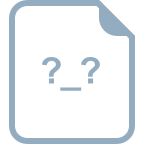
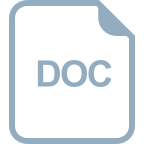
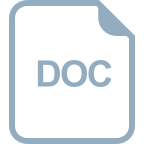
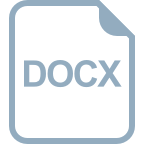
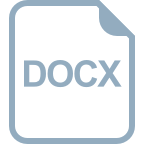
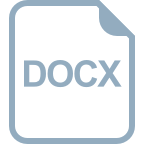