怎么用C语言读取一句英语中单词的个数
时间: 2024-10-19 11:07:13 浏览: 30
在C语言中,读取字符串中的单词个数需要将字符串按照空格或其他分隔符分割成单词数组,然后统计元素个数。以下是一个基本示例:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符的函数,如isspace()
int count_words(char *str) {
int word_count = 0;
int i = 0; // 字符索引
while (str[i]) { // 遍历字符串直到遇到结束符
if (isspace(str[i])) { // 如果遇到空格或其他空白字符
++i; // 跳过空格
continue;
}
if (!isspace(str[i + 1])) { // 检查下一个字符是否还是空格,如果不是,则开始一个新的单词计数
++word_count;
}
++i; // 继续遍历
}
if (str[i] == '\0') { // 如果最后一个字符是空字节,则说明这是一个单独的单词
++word_count;
}
return word_count;
}
int main() {
char sentence[] = "This is a sample sentence.";
int wordCount = count_words(sentence);
printf("The sentence has %d words.\n", wordCount);
return 0;
}
```
在这个例子中,`count_words` 函数会返回输入字符串中单词的数量。注意,这个函数假设单词是由空格分隔的,并且句子以空格结尾。如果要考虑其他分隔符或特殊情况,可能需要修改`isspace`的判断条件。
阅读全文
相关推荐









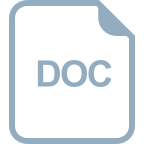

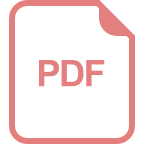


