收藏功能springboot+mybatisplus
时间: 2023-09-25 11:09:59 浏览: 66
收藏功能的实现可以分为以下几个步骤:
1. 数据库设计
首先需要设计一个收藏表,包含收藏ID、用户ID、收藏对象ID、创建时间等字段。
2. 实体类设计
根据数据库设计,创建一个对应的实体类,使用MyBatis Plus的注解标注表名、主键等信息。
3. Mapper接口设计
根据实体类设计一个对应的Mapper接口,继承MyBatis Plus的BaseMapper接口,即可直接调用MyBatis Plus提供的基本CRUD方法。
4. Service层实现
在Service层实现收藏功能的核心逻辑,主要包括添加收藏、删除收藏、查询收藏等操作。
5. Controller层实现
在Controller层实现接口,接收前端传来的请求参数,调用Service层的方法实现对应的功能,并返回结果给前端。
下面是一个简单的代码示例:
1. 数据库设计
```
CREATE TABLE `collection` (
`id` bigint(20) NOT NULL AUTO_INCREMENT COMMENT '收藏ID',
`user_id` bigint(20) NOT NULL COMMENT '用户ID',
`object_id` bigint(20) NOT NULL COMMENT '收藏对象ID',
`create_time` datetime NOT NULL COMMENT '创建时间',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COMMENT='收藏表';
```
2. 实体类设计
```
@Data
@TableName("collection")
public class Collection {
@TableId(type = IdType.AUTO)
private Long id;
private Long userId;
private Long objectId;
@TableField(fill = FieldFill.INSERT)
private Date createTime;
}
```
3. Mapper接口设计
```
public interface CollectionMapper extends BaseMapper<Collection> {
}
```
4. Service层实现
```
@Service
public class CollectionServiceImpl extends ServiceImpl<CollectionMapper, Collection> implements CollectionService {
@Override
public boolean addCollection(Long userId, Long objectId) {
Collection collection = new Collection();
collection.setUserId(userId);
collection.setObjectId(objectId);
return save(collection);
}
@Override
public boolean deleteCollection(Long collectionId) {
return removeById(collectionId);
}
@Override
public List<Collection> listCollections(Long userId) {
QueryWrapper<Collection> wrapper = new QueryWrapper<>();
wrapper.eq("user_id", userId);
return list(wrapper);
}
}
```
5. Controller层实现
```
@RestController
@RequestMapping("/collection")
public class CollectionController {
@Autowired
private CollectionService collectionService;
@PostMapping("/add")
public Result addCollection(@RequestParam Long userId, @RequestParam Long objectId) {
boolean result = collectionService.addCollection(userId, objectId);
return result ? Result.success() : Result.fail("添加收藏失败");
}
@PostMapping("/delete")
public Result deleteCollection(@RequestParam Long collectionId) {
boolean result = collectionService.deleteCollection(collectionId);
return result ? Result.success() : Result.fail("删除收藏失败");
}
@GetMapping("/list")
public Result listCollections(@RequestParam Long userId) {
List<Collection> collections = collectionService.listCollections(userId);
return Result.success(collections);
}
}
```
以上代码仅供参考,具体实现方式可以根据自己的需求进行调整。
相关推荐
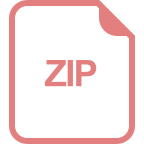
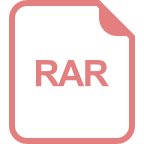
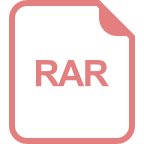















