int转换为unsigned char
时间: 2023-12-30 10:02:27 浏览: 24
可以使用C语言中的类型转换操作符将int类型转换为unsigned char类型,示例代码如下:
```
int num = 255;
unsigned char uchar_num = (unsigned char)num;
```
上述代码中,将int类型变量num的值转换为unsigned char类型,并赋值给变量uchar_num。请注意,这种类型转换可能会导致数据精度丢失,因此需要谨慎使用。
相关问题
unsigned int数组转化为 unsigned char 数组
可以通过循环遍历数组,将每个元素依次转化为 unsigned char 类型并存储在新的 unsigned char 数组中。例如以下代码可以实现该功能:
```
unsigned int myArray[] = {1, 2, 3, 4};
unsigned char newArray[sizeof(myArray)];
for (int i = 0; i < sizeof(myArray); i++) {
unsigned int value = myArray[i];
newArray[i * sizeof(unsigned int) + 0] = (value >> 24) & 0xFF;
newArray[i * sizeof(unsigned int) + 1] = (value >> 16) & 0xFF;
newArray[i * sizeof(unsigned int) + 2] = (value >> 8) & 0xFF;
newArray[i * sizeof(unsigned int) + 3] = value & 0xFF;
}
```
这段代码先定义了一个 unsigned int 数组 myArray 和一个新的 unsigned char 数组 newArray,然后通过循环遍历 myArray,将每个元素转化为 unsigned char 类型并存储在对应位置的 newArray 中。对于每个 unsigned int 元素,代码将它右移 24、16、8 和 0 位,然后使用位运算符“&”和“0xFF”提取出最低 8 位,即转化为 unsigned char 类型后的值。最后将这四个值存储在新数组中,其中每个元素占据了四个连续的位置。
unsigned int转unsigned char
### 回答1:
将unsigned int转换为unsigned char,可以使用强制类型转换。具体方法如下:
1. 定义一个unsigned int类型的变量,例如unsigned int num = 12345;
2. 定义一个unsigned char类型的变量,例如unsigned char ch;
3. 将num强制转换为unsigned char类型,赋值给ch,例如ch = (unsigned char)num;
4. 这样就完成了unsigned int到unsigned char的转换。
需要注意的是,如果unsigned int的值超过了unsigned char的范围(0~255),则转换后的结果可能会出现截断。
### 回答2:
unsigned int和unsigned char是不同的数据类型。unsigned int是无符号的整数类型,它通常占据4个字节(32位),可以表示的最大值为4294967295。而unsigned char是无符号的字符类型,它占据1个字节(8位),可以表示的最大值为255。在程序开发中,我们经常需要将unsigned int类型的变量转换为unsigned char类型,这可以通过类型转换实现。
将一个unsigned int类型的变量转换为unsigned char类型的变量时,要注意两个方面。首先,需要判断unsigned int类型变量的值是否在0到255之间,因为如果超出了unsigned char类型所能表示的范围,就会导致精度丢失。其次,需要考虑字节序(byte order)的影响。字节序指定计算机中多个字节值的存储顺序。在以小端模式(little-endian)存储的计算机中,最低有效字节(least significant byte)存储在最前面;而在以大端模式(big-endian)存储的计算机中,最高有效字节(most significant byte)存储在最前面。因此,在进行unsigned int转unsigned char类型的操作时,需要注意字节序对转换结果的影响。
以下是一个示例代码,演示了如何将unsigned int类型变量my_int转换为unsigned char类型变量my_char:
unsigned int my_int = 2105335039;
unsigned char my_char[4];
my_char[0] = (unsigned char)(my_int >> 0);
my_char[1] = (unsigned char)(my_int >> 8);
my_char[2] = (unsigned char)(my_int >> 16);
my_char[3] = (unsigned char)(my_int >> 24);
在这个例子中,先定义了一个unsigned int类型变量my_int,并初始化为一个非零值。然后定义了一个unsigned char类型的数组my_char,其长度为4字节,因为unsigned int类型数据也是4字节的。将my_int转化为unsigned char类型时,先将其右移0位、8位、16位和24位,在用无符号char的类型强制转换的方式取低8位,同时对应的赋值给my_char数组中的对应元素。这样,就完成了unsigned int类型转unsigned char类型的操作。要注意,这个转换过程中,若数据从低位转到高位的 , 会出现大小端的转换,需要根据实际情况来选择处理方式。
### 回答3:
在C/C++程序中,unsigned int和unsigned char是两种不同类型的数据类型,它们都是无符号数据类型,但它们的长度不同。unsigned int通常是32位的,而unsigned char通常是8位的。因此,如果我们想把一个unsigned int转换成unsigned char,就需要注意数据类型的不同以及它们的长度。
首先,我们需要明确的是,unsigned int和unsigned char之间的转换并不是一种精确的转换。因为unsigned int的长度远大于unsigned char,所以在将一个unsigned int转换成unsigned char的时候,会丢失一些高位数据,从而影响数据的精度。
如果我们想将一个unsigned int转换成unsigned char,可以使用C/C++的强制类型转换符号进行转换,具体方法如下:
unsigned int x = 300;
unsigned char y = (unsigned char)x;
在以上代码中,将一个整型常量300赋值给unsigned int类型的变量x,然后使用强制类型转换符号将x转换为unsigned char类型的变量y,最终y的值为44。
需要注意的是,如果在转换过程中,unsigned int类型的变量x的值超过了unsigned char类型的最大值255,那么转换后的值将被截取为unsigned char类型的最大值255。同样地,如果x的值小于unsigned char类型的最小值0,那么转换后的值将被截取为unsigned char类型的最小值0。
总之,在进行unsigned int到unsigned char的转换时,需要注意类型的差异及其长度不同所带来的数据损失问题。在实际操作中,需要结合具体的场景和需求来进行类型转换,并做好数据精度的控制和保障,以避免出现数据错误及逻辑错误的情况。
相关推荐
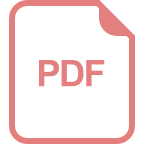
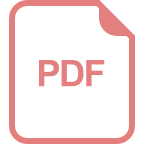
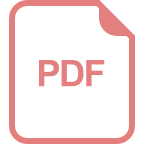












