帮我写一个C#编程的卡拉ok点歌系统
时间: 2024-03-10 11:47:30 浏览: 141
好的,我可以为您提供一个基本的卡拉OK点歌系统的C#编程代码。以下是一个简单的实现:
```csharp
using System;
using System.Collections.Generic;
namespace KaraokeSystem
{
class Song
{
public string Title { get; set; }
public string Artist { get; set; }
public string Genre { get; set; }
public int Year { get; set; }
}
class KaraokeMachine
{
private List<Song> songs = new List<Song>();
public void AddSong(Song song)
{
songs.Add(song);
}
public void RemoveSong(Song song)
{
songs.Remove(song);
}
public List<Song> GetAllSongs()
{
return songs;
}
public List<Song> SearchSongs(string searchTerm)
{
List<Song> results = new List<Song>();
foreach (Song song in songs)
{
if (song.Title.ToLower().Contains(searchTerm.ToLower()) || song.Artist.ToLower().Contains(searchTerm.ToLower()))
{
results.Add(song);
}
}
return results;
}
}
class Program
{
static void Main(string[] args)
{
KaraokeMachine machine = new KaraokeMachine();
// Add sample songs
machine.AddSong(new Song { Title = "Shape of You", Artist = "Ed Sheeran", Genre = "Pop", Year = 2017 });
machine.AddSong(new Song { Title = "Bohemian Rhapsody", Artist = "Queen", Genre = "Rock", Year = 1975 });
machine.AddSong(new Song { Title = "Billie Jean", Artist = "Michael Jackson", Genre = "Pop", Year = 1983 });
machine.AddSong(new Song { Title = "Yesterday", Artist = "The Beatles", Genre = "Rock", Year = 1965 });
bool running = true;
while (running)
{
Console.WriteLine("Welcome to the Karaoke Machine!");
Console.WriteLine("1 - Show all songs");
Console.WriteLine("2 - Search for a song");
Console.WriteLine("3 - Add a song");
Console.WriteLine("4 - Remove a song");
Console.WriteLine("5 - Quit");
string choice = Console.ReadLine();
switch (choice)
{
case "1":
List<Song> allSongs = machine.GetAllSongs();
Console.WriteLine("All songs:");
foreach (Song song in allSongs)
{
Console.WriteLine($"{song.Title} by {song.Artist} ({song.Genre}, {song.Year})");
}
break;
case "2":
Console.WriteLine("Enter search term:");
string searchTerm = Console.ReadLine();
List<Song> searchResults = machine.SearchSongs(searchTerm);
Console.WriteLine($"Search results for '{searchTerm}':");
foreach (Song song in searchResults)
{
Console.WriteLine($"{song.Title} by {song.Artist} ({song.Genre}, {song.Year})");
}
break;
case "3":
Console.WriteLine("Enter song title:");
string title = Console.ReadLine();
Console.WriteLine("Enter song artist:");
string artist = Console.ReadLine();
Console.WriteLine("Enter song genre:");
string genre = Console.ReadLine();
Console.WriteLine("Enter song year:");
int year = int.Parse(Console.ReadLine());
machine.AddSong(new Song { Title = title, Artist = artist, Genre = genre, Year = year });
Console.WriteLine("Song added!");
break;
case "4":
Console.WriteLine("Enter song title to remove:");
string titleToRemove = Console.ReadLine();
List<Song> songsToRemove = machine.SearchSongs(titleToRemove);
if (songsToRemove.Count > 0)
{
machine.RemoveSong(songsToRemove[0]);
Console.WriteLine($"{titleToRemove} removed!");
}
else
{
Console.WriteLine($"Song '{titleToRemove}' not found.");
}
break;
case "5":
running = false;
Console.WriteLine("Goodbye!");
break;
default:
Console.WriteLine("Invalid choice.");
break;
}
Console.WriteLine();
}
}
}
}
```
这个程序包含了一个 `Song` 类来表示歌曲,一个 `KaraokeMachine` 类来管理歌曲,并且在主程序中提供了一个简单的用户界面来让用户选择不同的选项。用户可以查看所有的歌曲、搜索歌曲、添加歌曲和删除歌曲。
阅读全文
相关推荐



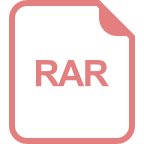
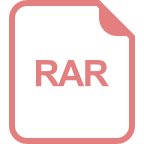
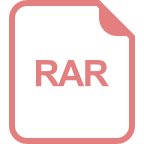


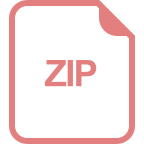
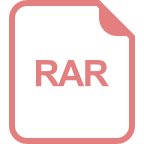




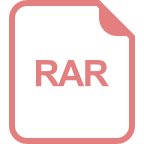
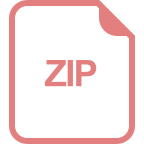
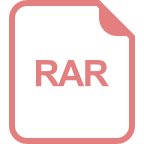
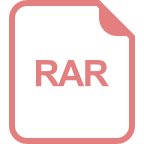