c++读写带逗号分隔符的txt点坐标的文件
时间: 2024-10-13 22:13:18 浏览: 29
在C++中,处理包含逗号分隔值(CSV)的点坐标的文本文件非常常见。可以使用标准库中的`fstream`类来完成读写操作。这里有一个基本的例子,展示了如何读取和写入这样的文件:
```cpp
#include <fstream>
#include <sstream>
#include <vector>
#include <stdexcept>
// 点类定义
class Point {
public:
double x, y, z;
Point(double x, double y, double z) : x(x), y(y), z(z) {}
};
// 读取CSV文件
std::vector<Point> read_csv(const std::string& filename) {
std::ifstream input_file(filename);
if (!input_file.is_open()) {
throw std::runtime_error("无法打开文件:" + filename);
}
std::vector<Point> points;
std::string line;
while (std::getline(input_file, line)) {
if (line.empty() || line[0] == '#') continue; // 忽略空行和注释
std::istringstream iss(line);
Point p;
if (!(iss >> p.x >> p.y >> p.z)) { // 如果解析失败,跳过此行
std::cerr << "警告:错误解析行" << line << std::endl;
continue;
}
points.push_back(p);
}
input_file.close();
return points;
}
// 写入CSV文件
void write_csv(const std::vector<Point>& points, const std::string& filename) {
std::ofstream output_file(filename);
if (!output_file.is_open()) {
throw std::runtime_error("无法创建文件:" + filename);
}
for (const auto& point : points) {
output_file << point.x << "," << point.y << "," << point.z << "\n"; // 每个点一行并用逗号分隔
}
output_file.close();
}
int main() {
try {
std::vector<Point> point_cloud = read_csv("input.csv");
write_csv(point_cloud, "output.csv");
std::cout << "成功处理文件!" << std::endl;
} catch (const std::exception& e) {
std::cerr << "错误:" << e.what() << std::endl;
}
return 0;
}
```
这个示例中,`read_csv`函数读取文件并将每行转换为`Point`对象,`write_csv`函数则是将`Point`对象列表写回文件。注意在读取时我们检查了解析的成功与否,若遇到格式问题会忽略该行。
阅读全文
相关推荐
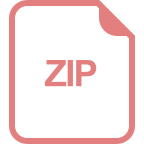
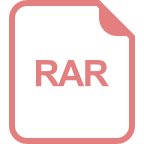
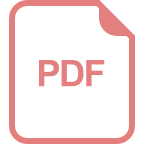
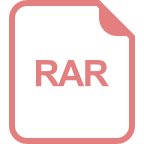
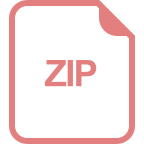
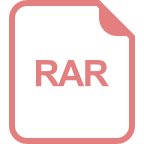
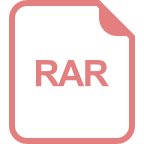
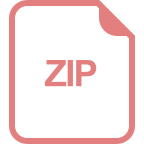
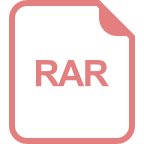
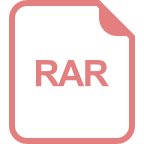
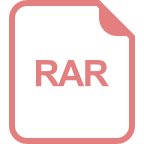
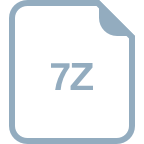
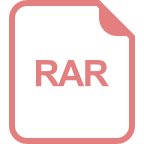
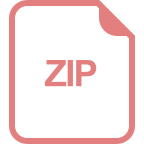
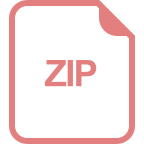
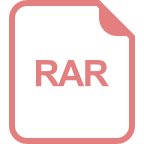
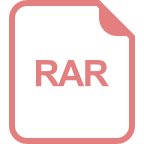
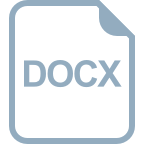